MUTATIONS¶
It will be a function used to write data or change data in the database and can respond with the same results as Queries. It consists of:
ClearFeed¶
To delete all data in the Time Series Data Store of that device. Use in cases where you want to delete data during system testing or data that is not actually used. Details are as follows:
clearFeed ( feedid ):
Arguments
feedid
: String is the Feed ID which has the same value asdeviceid
(required).
Query Variables
Authorization
: User Token
Response Type : FeedData
The response is Object Type FeedData consisting of:
feedid
: String is the Feed ID.
data
: JSON is the data in each field.
An example is shown in the following figure:
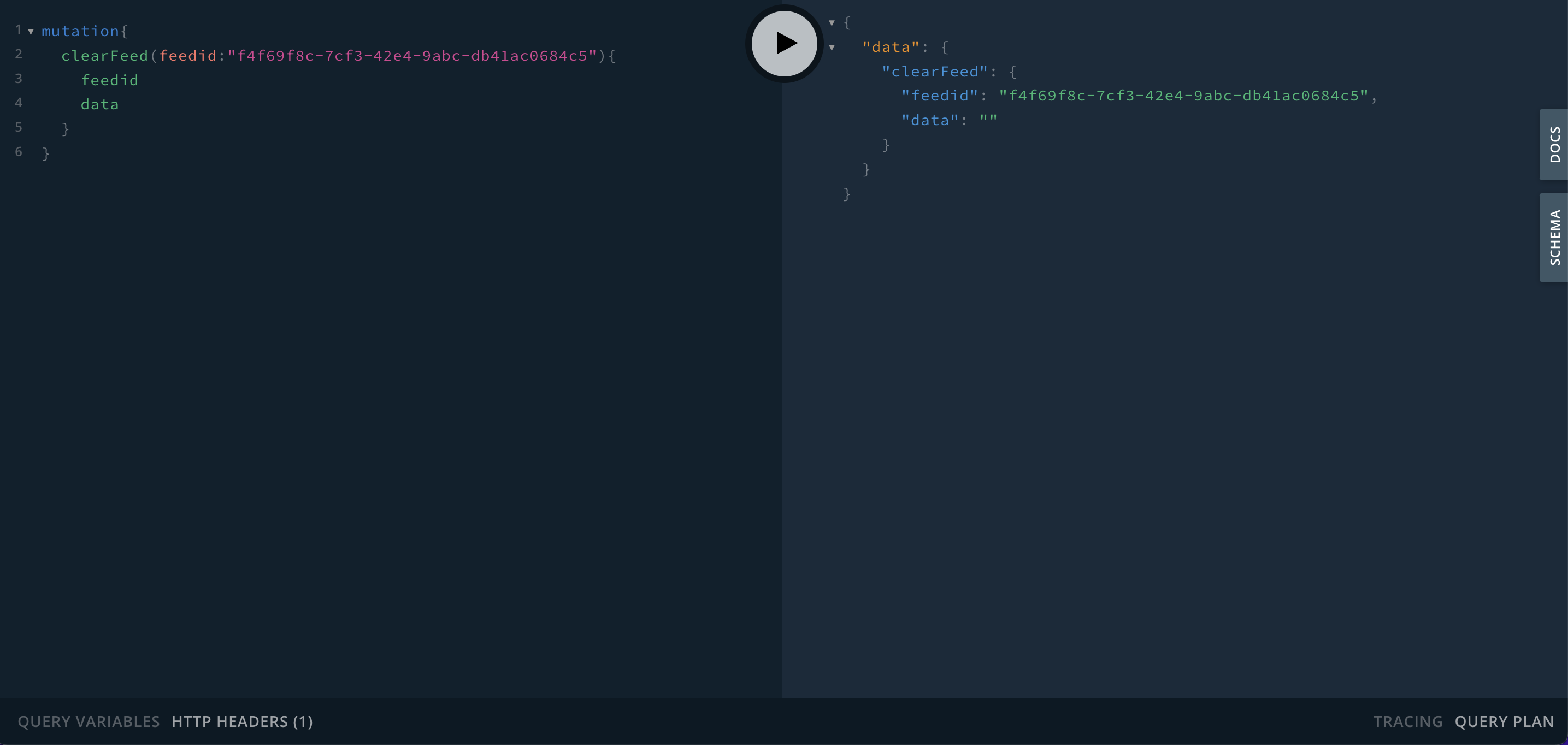
CreateAPIToken¶
For creating and requesting API Tokens as Authentication to use various APIs in the Platform, details are as follows:
createAPIToken ( payload, nbf, expireIn ):
Arguments
payload
: payload is specifying what is allowed to be accessed in Object Type format, separated into 3 fields:
device
: [device_permission] is the permission to access the Device in the form of an Array of Objects consisting of:
deviceid
: String is the Device ID that is allowed to be accessed.
scope
: [scope] is the scope of access rights, including:
read_shadow
(Allow reading of Device Shadow)
write_shadow
(Allow writing of Device Shadow)
read_feed
(Allow reading of data in the Device's Timeseries Data)
read_devicestatus
(Allow reading the Device’s connection status to the platform)
project
: [project_permission] is the permission to access the Project in the form of an Array of Objects consisting of:
projectid
: String is the Project ID that allows access.
scope
: [scope] is the scope of access rights, including:
read_shadow
(Allow reading of Shadow of all Devices under the Project)
write_shadow
(Allow writing of Shadow of all Devices under the Project)
read_feed
(Allow reading of data in Timeseries Data of all devices under the Project)
read_devicestatus
(Allow reading the Device’s connection status to the platform of all devices under the project)
group
: [group_permission] is the access rights to the Group in the form of an Array of Objects consisting of:
groupid
: String is the ID of the Group that is allowed to access.
scope
: [scope] is the scope of access rights, including:
read_shadow
(Allow reading of Shadow of all Devices under the Group)
write_shadow
(Allow writing of Shadow of all Devices under the Group)
read_feed
(Allow reading of data in Timeseries Data of all devices under the Group)
read_devicestatus
(Allow reading the Device’s connection status to the platform of all devices under the Group)
nbf
: nbf_format is the time when the token will be available. It is in the format of Object Type { unit: value }. It can specify 3 units of value:
minutes
: Int is the duration in minutes.
hours
: Int is the duration in hours.
days
: Int is the duration in days.
expireIn
: expireIn_format is the age of the Token in the format of Object Type { unit: value }. It can specify 3 units of value:
minutes
: Int is the duration in minutes.
hours
: Int is the duration in hours.
days
: Int is the duration in days.
Query Variables
Authorization
: User Token
Response Type : ApiToken
The response is an Object Type ApiToken consisting of:
access_token
: String is a token for accessing various API services according to the rights granted.
refresh_token
: String is a Token used to request a new Access Token when it expires.
iat
: String is the date and time the token was issued (issued at).
nbf
: String is the date when the token will be active (not before).
exp
: String is the expiration time of the Token.
expireIn
: String is the age of the Token.
userid
:String is the user code that grants the permission.
_key
:String is a reference code.
An example is shown in the following figure:
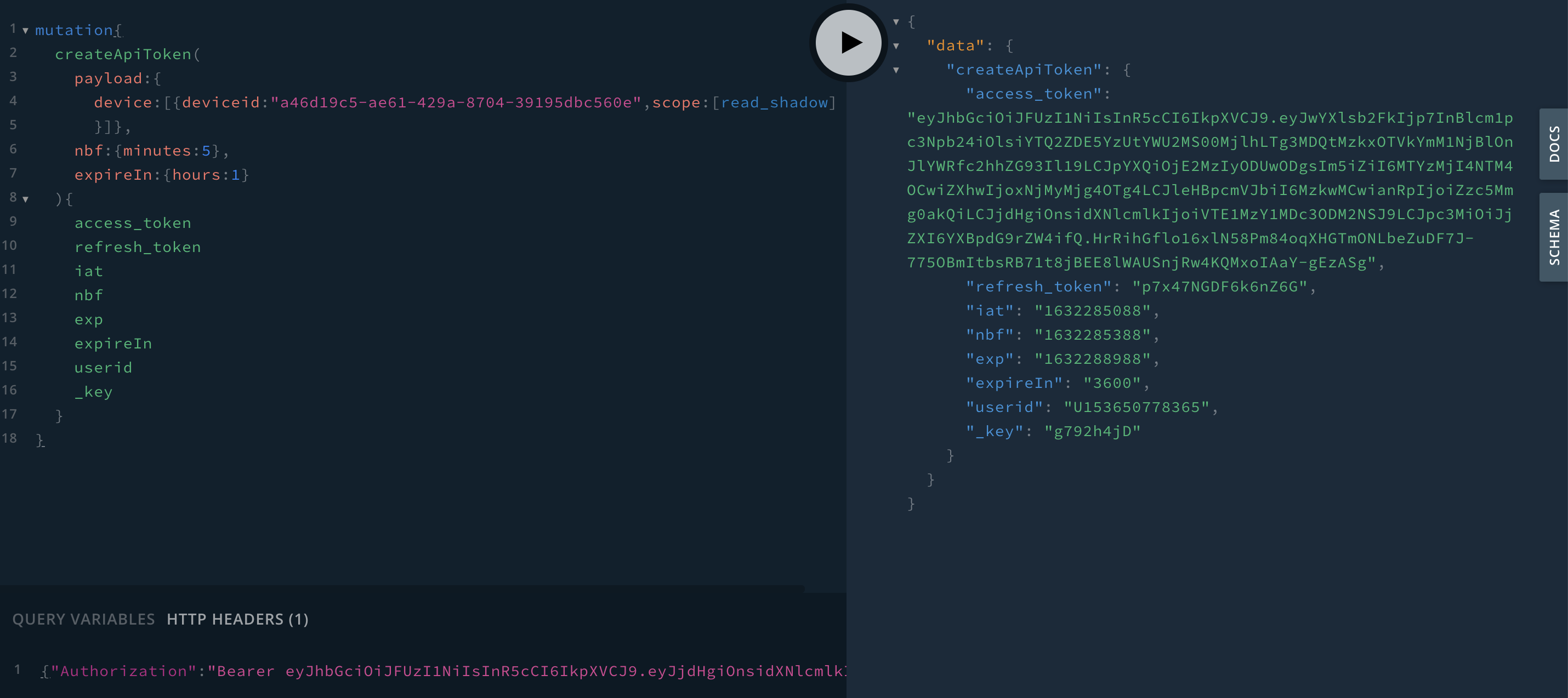
CreateDevice¶
To request to create a new device, the details are as follows:
createDevice ( projectid, deviceid, deviceinfo ):
Arguments
projectid
: String is the Project ID that the Device will be under (required)
deviceid
: String is the Device ID (if not specified, the system will automatically generate it)
deviceinfo
: DeviceInfo is an Object Type that contains details about the Device, including:
alias
: String is the name of the Device (if not specified it will be set to the same value as deviceid, used to refer to the Device).
description
: String is a Device description.
tag
: String is a Device tag in single value format.
hashtag
: [String] is the Device tag in multi-value format.
tags
: JSON is a Device tag in Key Value format.
Query Variables
Authorization
: User Token
Response Type : DeviceWithToken
The response is of Object Type DeviceWithToken consisting of:
alias
: String is the name of the Device.
createdtime
: Timestamp is the date and time the Device was created.
credential
: Credential is data for verifying identity. Object Type credential consists of:
secret
: String is the Device's secret code for Authentication.
description
: String is a Device description.
deviceid
: String the Device ID.
devicetoken
: [String] is a list of all tokens of the Device.
devicesecret
: String is the secret code of the Device.
groupid
: String is the ID of the Group that the Device is under.
groupname
: String is the name of the Group that the Device is under.
projectid
: String is the Project ID that the Device is under.
status
: Int is Device’s connection status to the platform (0
offline,1
online)
tag
: String is a Device tag in single value format.
hashtag
: [String] is the Device tag in multi-value format.
tags
: JSON is a Device tag in Key Value format.
enabled
: Boolean is the Device activation status (true
enabled [default],false
disabled).
banned
: Boolean is the status of being suspended from the system (true
suspended,false
not suspended [default]).
token
: Token is an Object Type of Token data. It consists of:
tokencode
: String is the code of the Token.
iat
: String is the date and time the token was issued (issued at).
nbf
: String is the date when the token will be active (not before).
exp
: String is the expiration time of the Token.
expireIn
: String is the age of the Token.
An example is shown in the following figure:
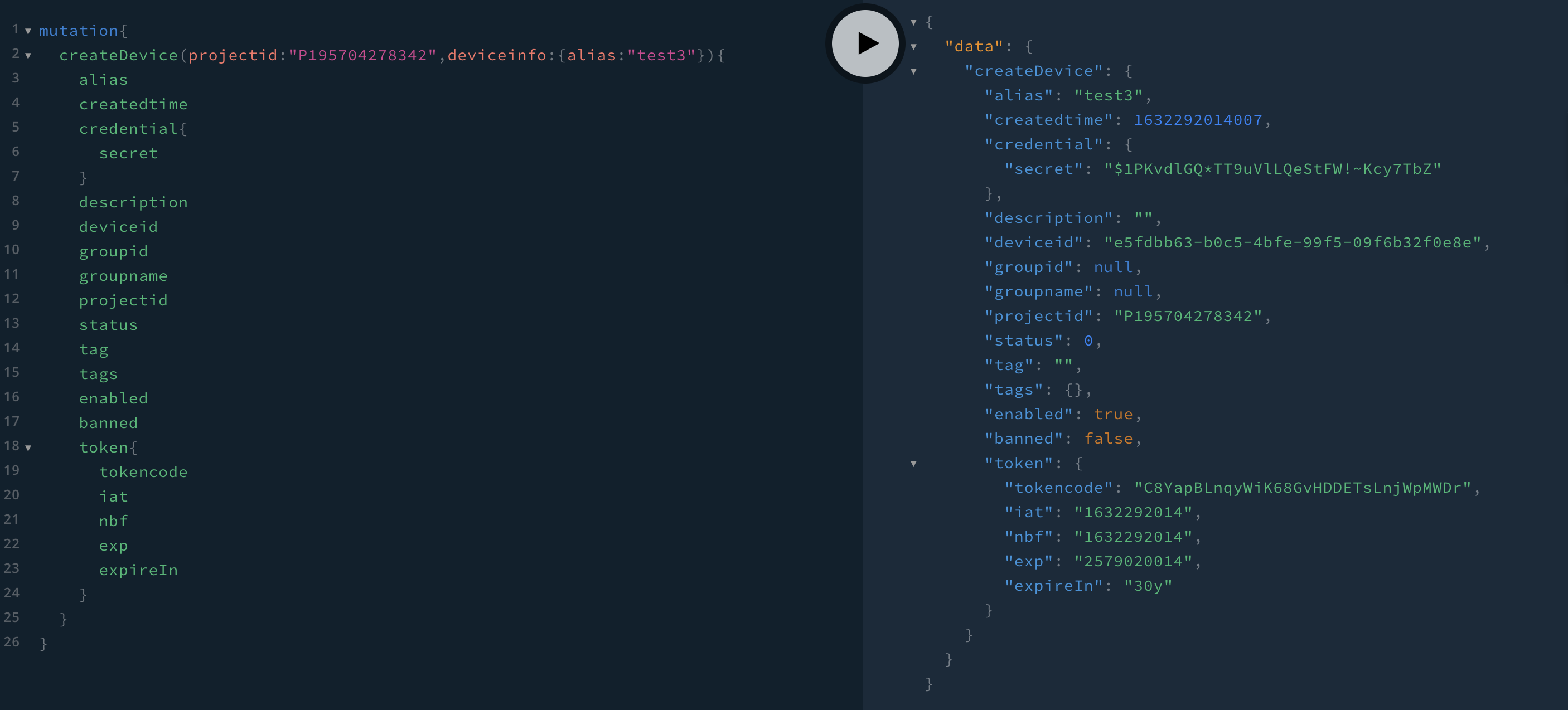
CreateDeviceToken¶
To request to create a new Device Token, the details are as follows:
createDeviceToken ( deviceid, expireIn, nbf, scope, verify ):
Arguments
deviceid
: String is the Device ID (required)
expireIn
: String is the age of the Token.
nbf
: String is the date when the token will be active (not before).
scope
: [String] is the scope of the Token that can be operated on. It will be in the form of an Array of Strings.
verify
: Boolean is the submitted data must be checked for correctness.
Query Variables
Authorization
: User Token
Response Type : DeviceToken
The response is an Object Type DeviceToken consisting of:
deviceid
: String is the Device ID.
tokencode
: String is the code of the Token.
iat
: String is the date and time the token was issued (issued at).
nbf
: String is the date when the token will be active (not before).
exp
: String is the expiration time of the Token.
expireIn
: String is the age of the Token.
An example is shown in the following figure:
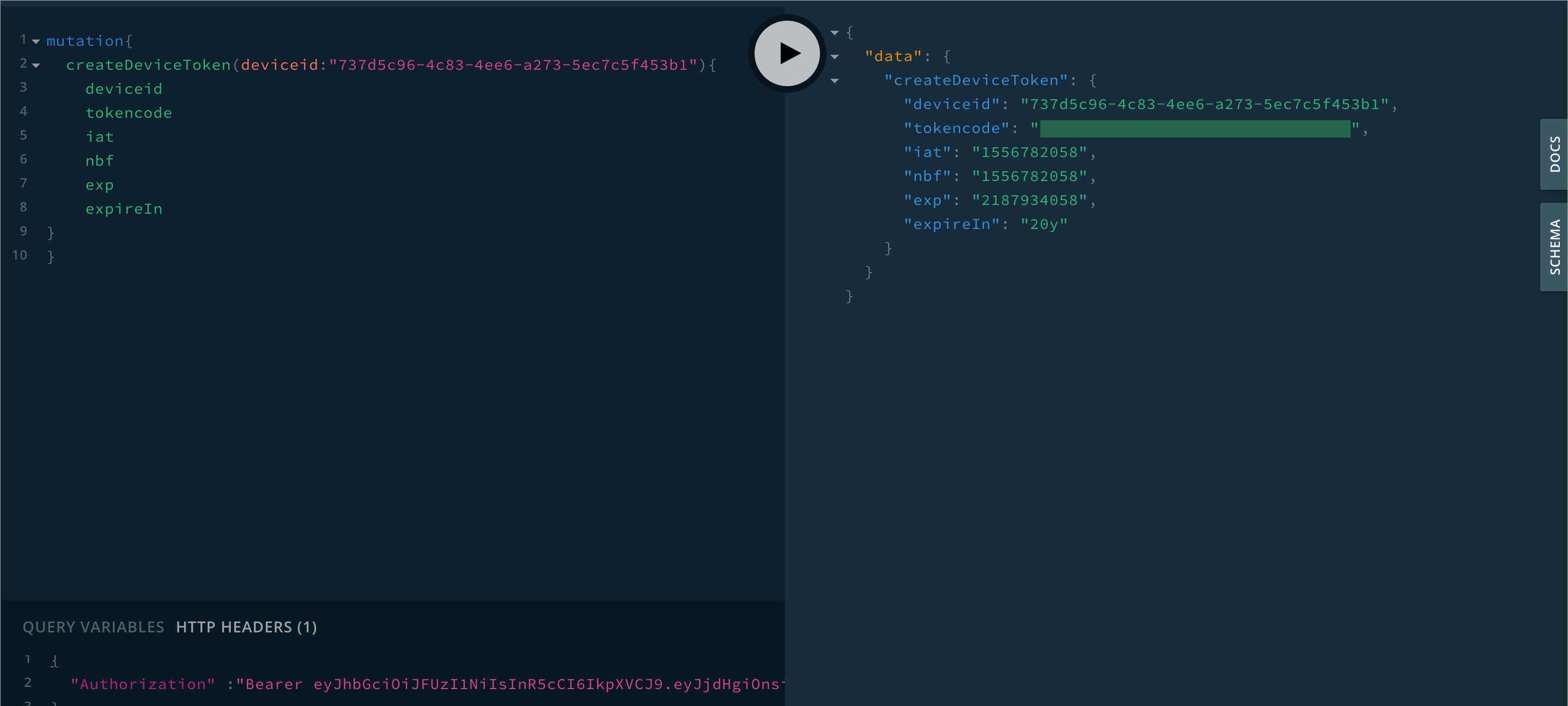
CreateGroup¶
To request the creation of a new Group, the details are as follows:
createGroup ( projectid, groupname, description, tag, hashtag, tags ):
Arguments
projectid
: String is the Project ID that the Group is under (required)
groupname
: String is the name of the Group.
description
: String is a description of the Group.
tag
: String is a single-valued Group tag.
hashtag
: [String] is a multi-valued Group tag.
tags
: JSON is a Group tag in Key Value format.
Query Variables
Authorization
: User Token
Response Type : Group
The response is an Object Type Group consisting of:
groupid
: String is the ID of the Group.
groupname
: String is the name of the Group.
projectid
: String is the Project ID.
createdtime
: Timestamp is the date and time the Group was created.
description
: String is a description of the Group.
tag
: String is a single-valued Group tag.
hashtag
: [String] is a multi-valued Group tag.
tags
: Json is a Group tag in Key Value format.
An example is shown in the following figure:
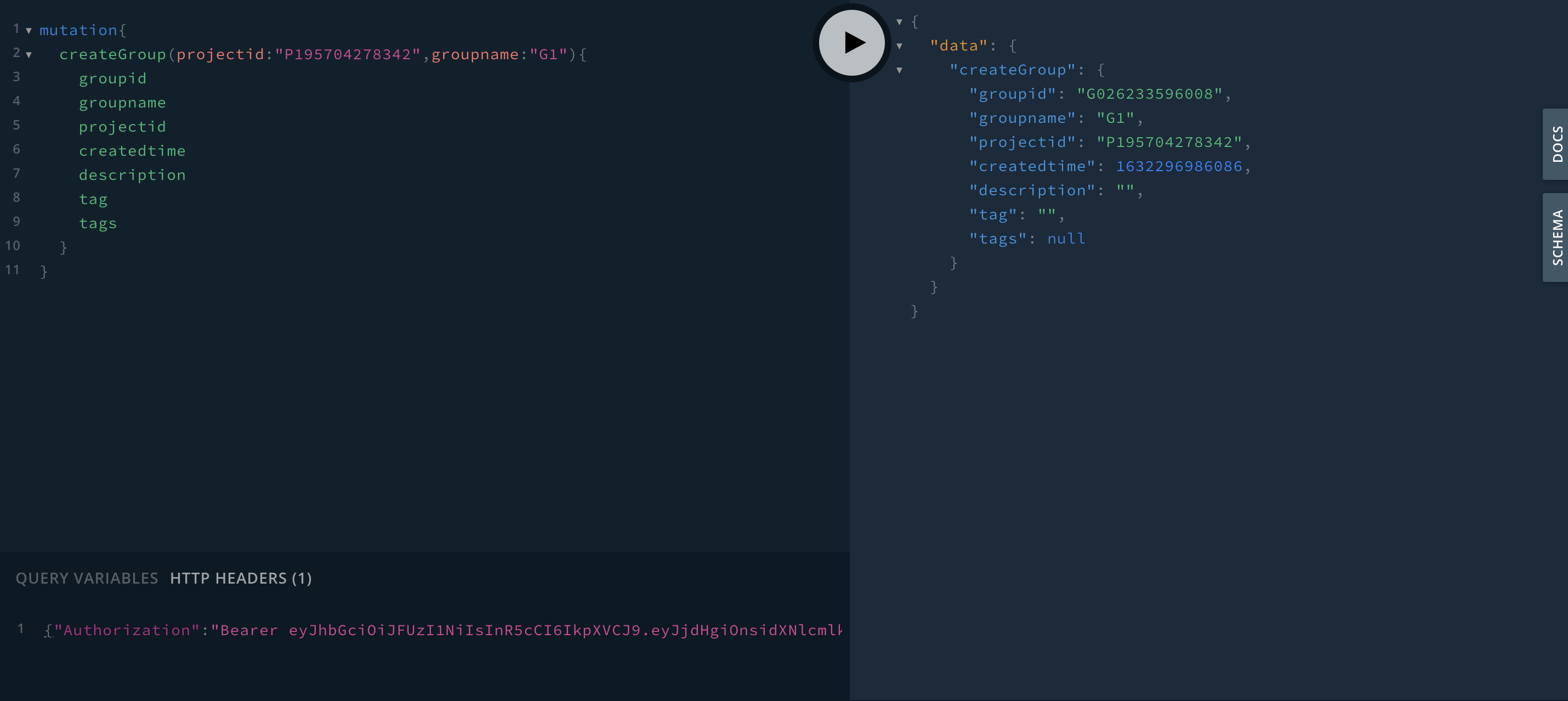
CreateHook¶
To create a new Event Hook, the details are as follows:
createHook ( projectid, name, description, type, enabled, param ):
Arguments
projectid: String is the Project ID (required).
name: String is the name of the Hook (required).
description: String is the description of the Hook.
type: String is the type of Hook (currently there is only one type: WEBHOOK)
enabled
: Boolean is the state of the Hook's activation (true
enabled,false
disabled [default]).param: JSON are the parameters set in the Hook.
Query Variables
Authorization
: User Token
Response Type : Hook
The response is an Object Type Hook consisting of:
projectid
: String is the Project ID.
name
: String is the name of the Hook.
hookid
: String is the code of Hook.
description
: String is a description of Hook.
type
: String is a type of Hook.
enabled
: Boolean is the state of the Hook (true
enabled,false
disabled).
param
: JSON is the various parameters set in the Hook.
An example is shown in the following figure:
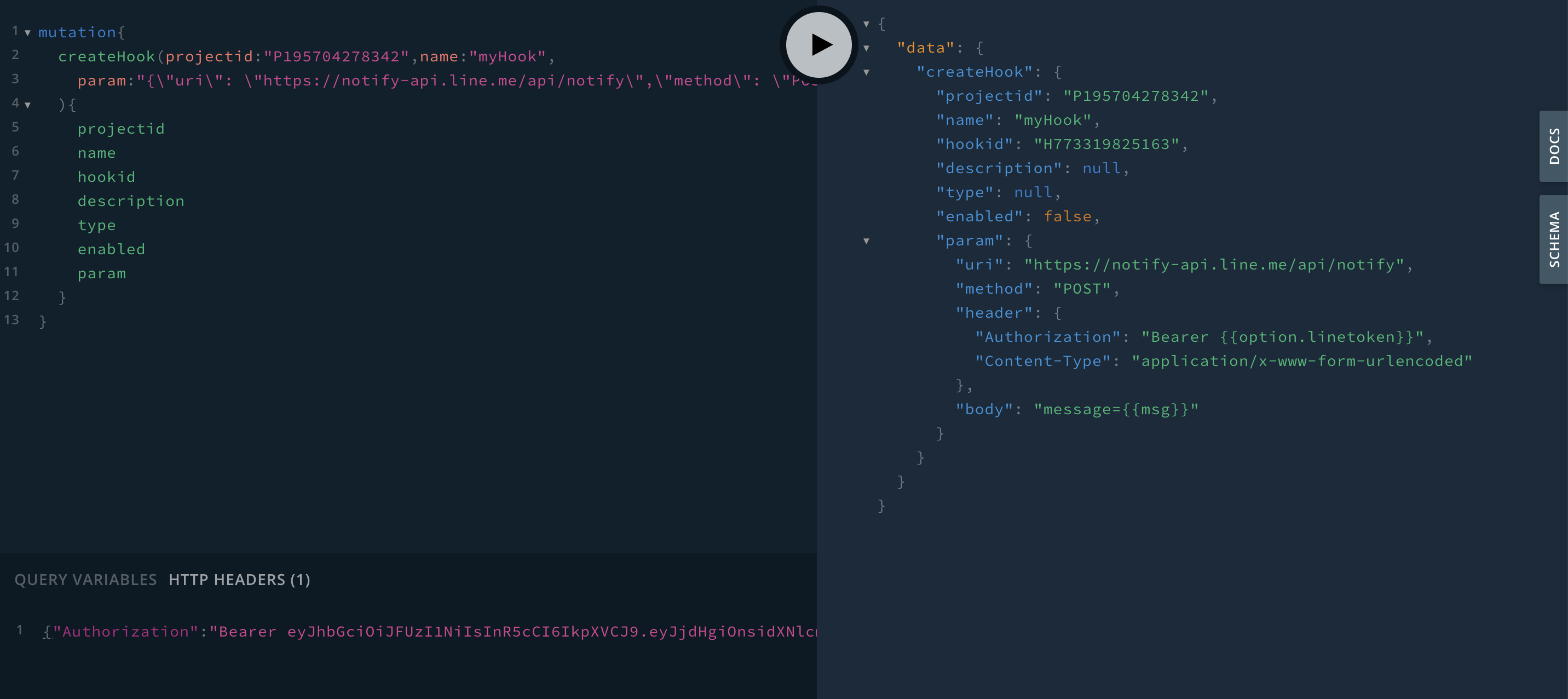
CreateMembership¶
To create a new Membership that has access to data in each Project that you do not own, the details are as follows:
createMembership ( projectid, username, level ):
Arguments
projectid
: String the Project ID (required)
username
: String is the username of the member user (required).
level
: UserLevel is the membership level, including (for details on rights, see Member)
Guest
(default)
Viewer
Editor
Master
Owner
Query Variables
Authorization
: User Token
Response Type : Membership
Response is Object Type Membership consists of
createdtime
: Timestamp is the date and time the Membership was created.
level
: UserLevel is the level of membership privileges. There are 5 levels in total (for details of privileges, see Member)
5
(Owner)
4
(Master)
3
(Editor)
2
(Viewer)
1
(Guest)
projectid
: String is the ID of the authorized project.
userid
: String is the user ID that is authorized.
username
: String is the username of the authorized user.
An example is shown in the following figure:
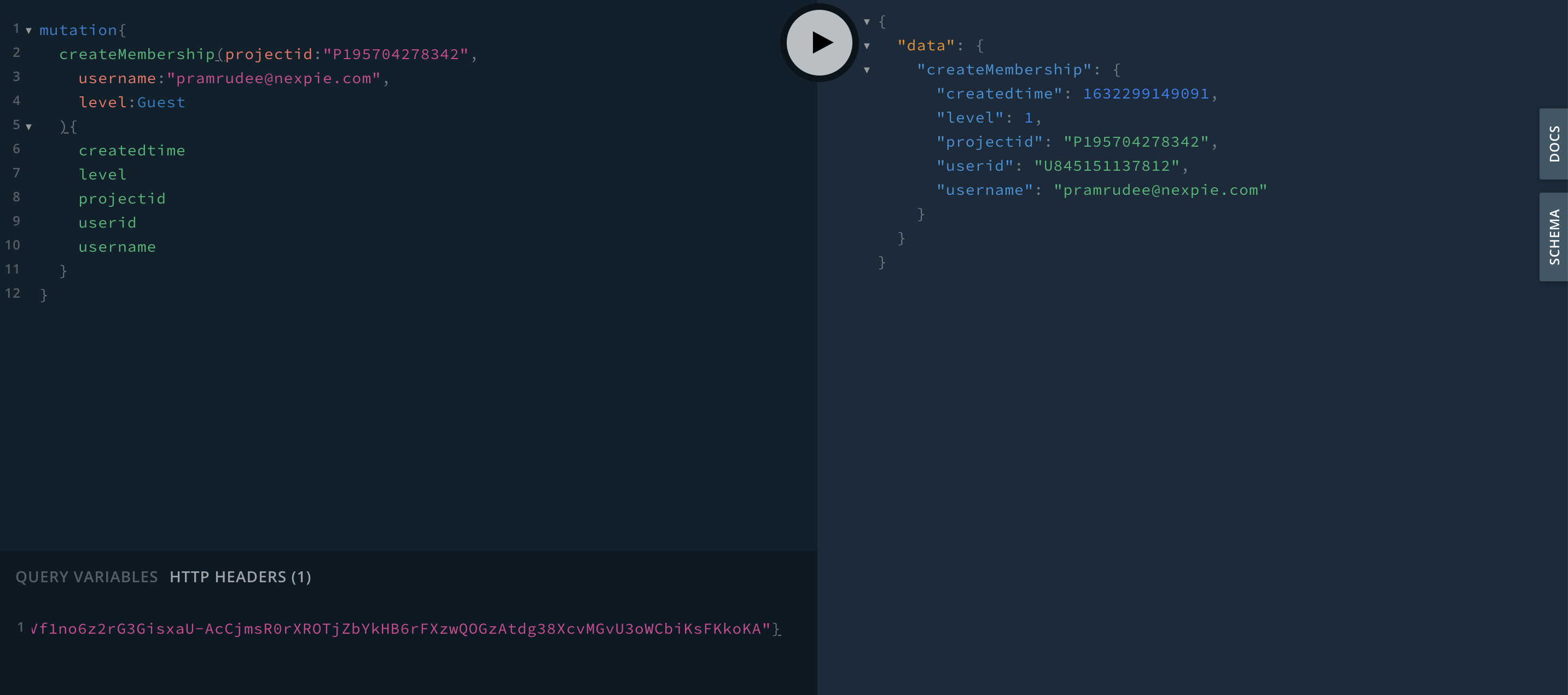
CreateProject¶
To create a new project, the details are as follows:
createProject ( projectname, billingaccountid, description, tag, hashtag, tags ):
Arguments
projectname
: String is the name of the Project (required)
billingaccountid
: String is the Billing Account ID.
description
: String is a project description.
tag
: String is a single-valued Project tag.
hashtag
: [String] is a multi-valued Project tag.
tags
: TagsInput is the project tags in Key Value format.
Query Variables
Authorization
: User Token
Response Type : Project
The response is Object Type Project consisting of:
projectname
: String is the name of the Project.
projectid
: String is the Project ID.
createdtime
: Timestamp is the date and time the project was created.
description
: String is a project description.
userlevel
: String is the user's project access level.
tags
: Json is a project tag in Key Value format.
tag
: String is a single-valued Project tag.
hashtag
: [String] is a multi-valued Project tag.
numberdevice
: Int is the number of devices under the project.
numbergroup
: Int is the number of Groups under the Project.
numberdeviceonline
: Int is the number of devices currently connected to the Platform under the Project.
numberdeviceoffline
: Int is the number of Devices currently not connected to the Platform under the Project.
quota
: Quota is the status of each service with available quota, which includes:
apicall
: Boolean is a REST API service (true
available quota,false
unavailable or no quota)
connection
: Boolean คือ บริการเชื่อมต่อ Platform ของ Device (true
available quota,false
unavailable or no quota).
message
: Boolean is a Real Time Message service via MQTT Protocol (true
available quota,false
unavailable or no quota).
shadowops
: Boolean is a Shadow read/write service (true
available quota,false
unavailable or no quota)
store
: Boolean is a service that stores data in Time Series Data (true
available quota,false
unavailable or no quota)
trigger
: Boolean is Trigger & Action (Notification) service (true
available quota,false
unavailable or no quota)
datasource
: Boolean is the sum of the Byte size of the data that has been used by theapicall
service (true
available quota,false
unavailable or no quota)
An example is shown in the following figure:
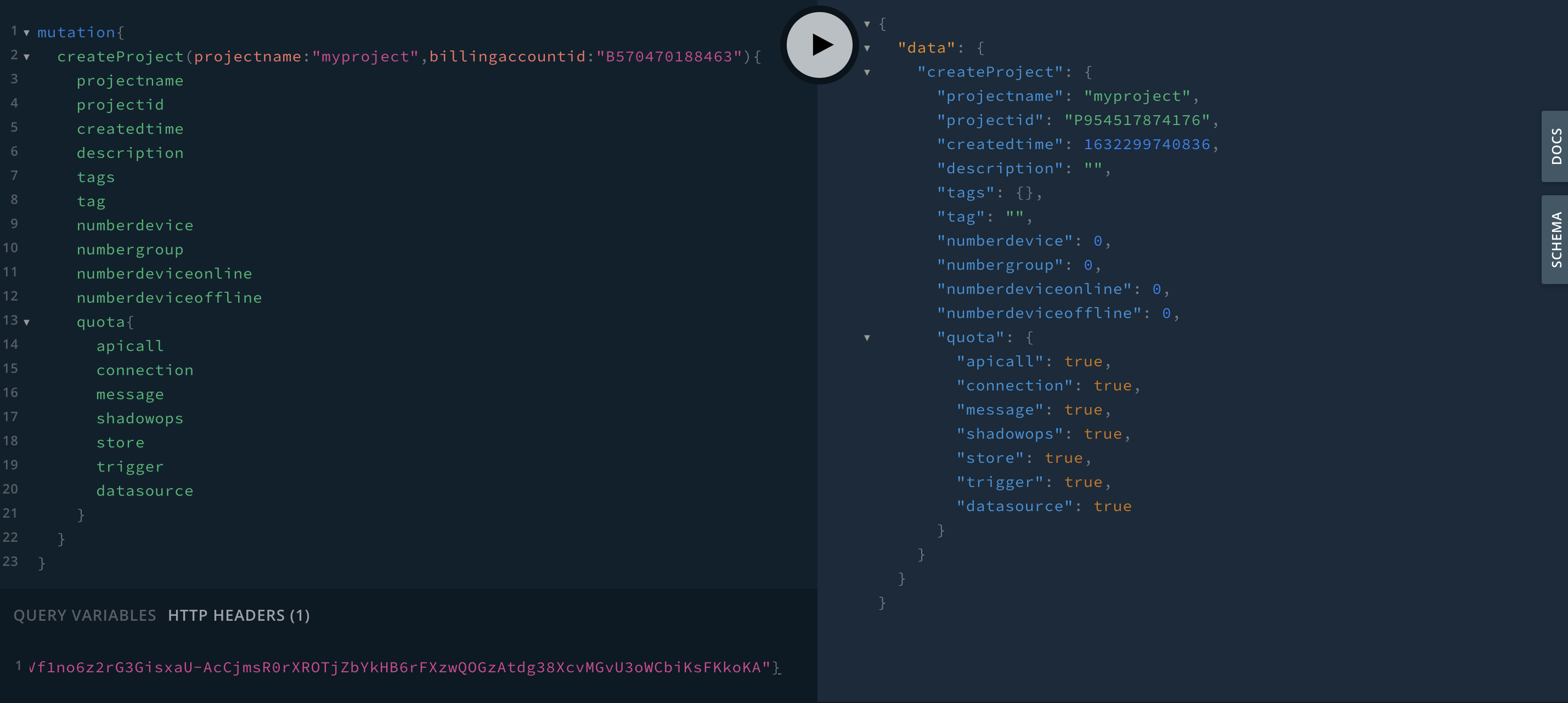
CreateTrigger¶
To create Device Trigger data, the details are as follows:
createTrigger ( deviceid, triggerid, triggername, type, event, condition, action, context, enabled ):
Arguments
deviceid
: String is the Device ID (required)
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: TriggerType is Trigger type (DEVICE
,SHADOW
) (required)
event
: String คือ is the event that triggered (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
) (required)
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook. It consists of:
key
: String is the name of the variable.
value
: String is the value of the variable.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
Query Variables
Authorization
: User Token
Response Type : [Trigger]
The response is an Array of Object Type Triggers consisting of:
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String คือ is the event that triggered (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
) (required)
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
An example is shown in the following figure:
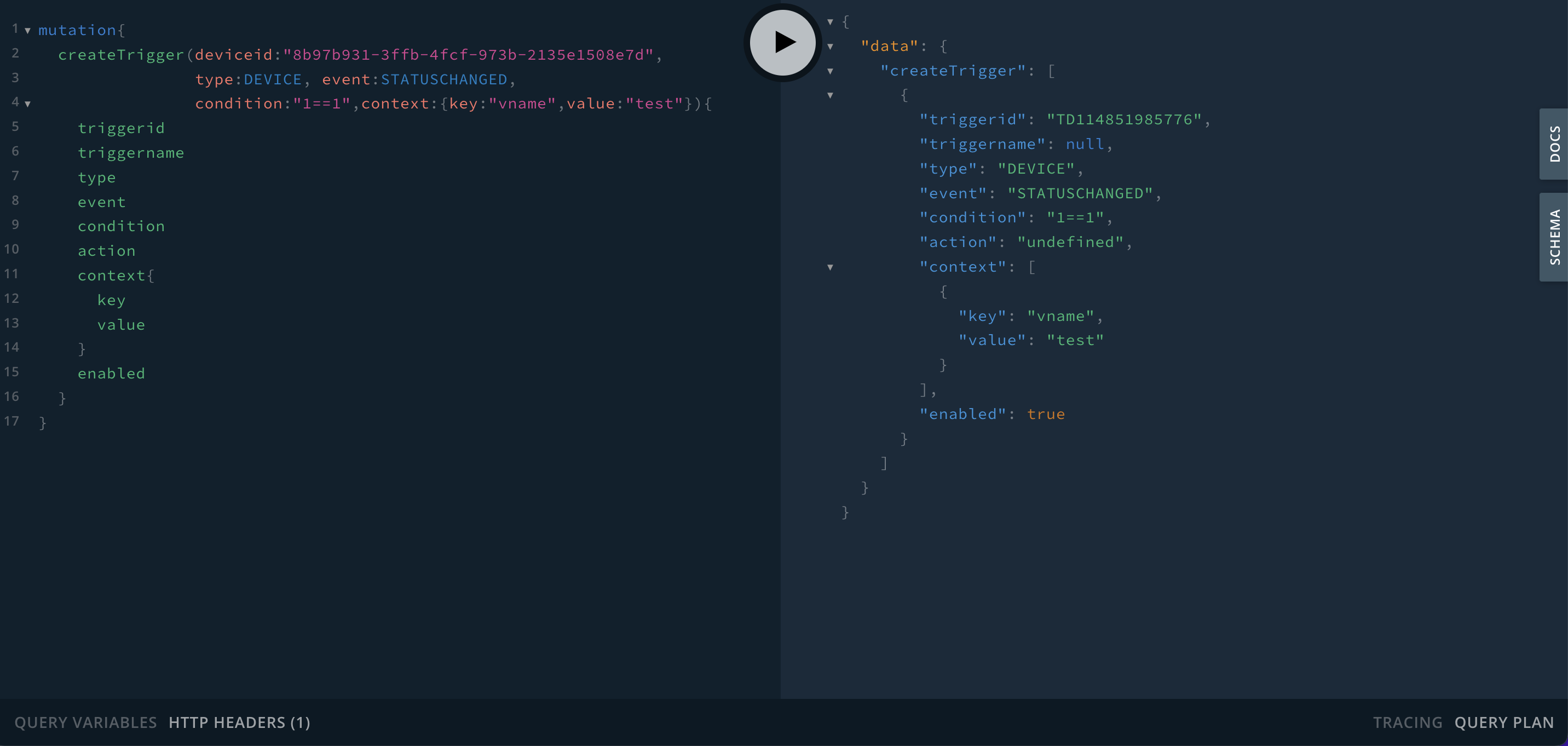
DeleteAllTriggers¶
To delete all Trigger data of the Device, details are as follows:
deleteAllTriggers ( deviceid ):
Arguments
deviceid
: String is the Device ID for which the Trigger is to be removed (required).
Query Variables
Authorization
: User Token
Response Type : [Trigger]
The response is an Array of Object Type Triggers consisting of:
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String คือ is the event that triggered (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
) (required)
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
An example is shown in the following figure:
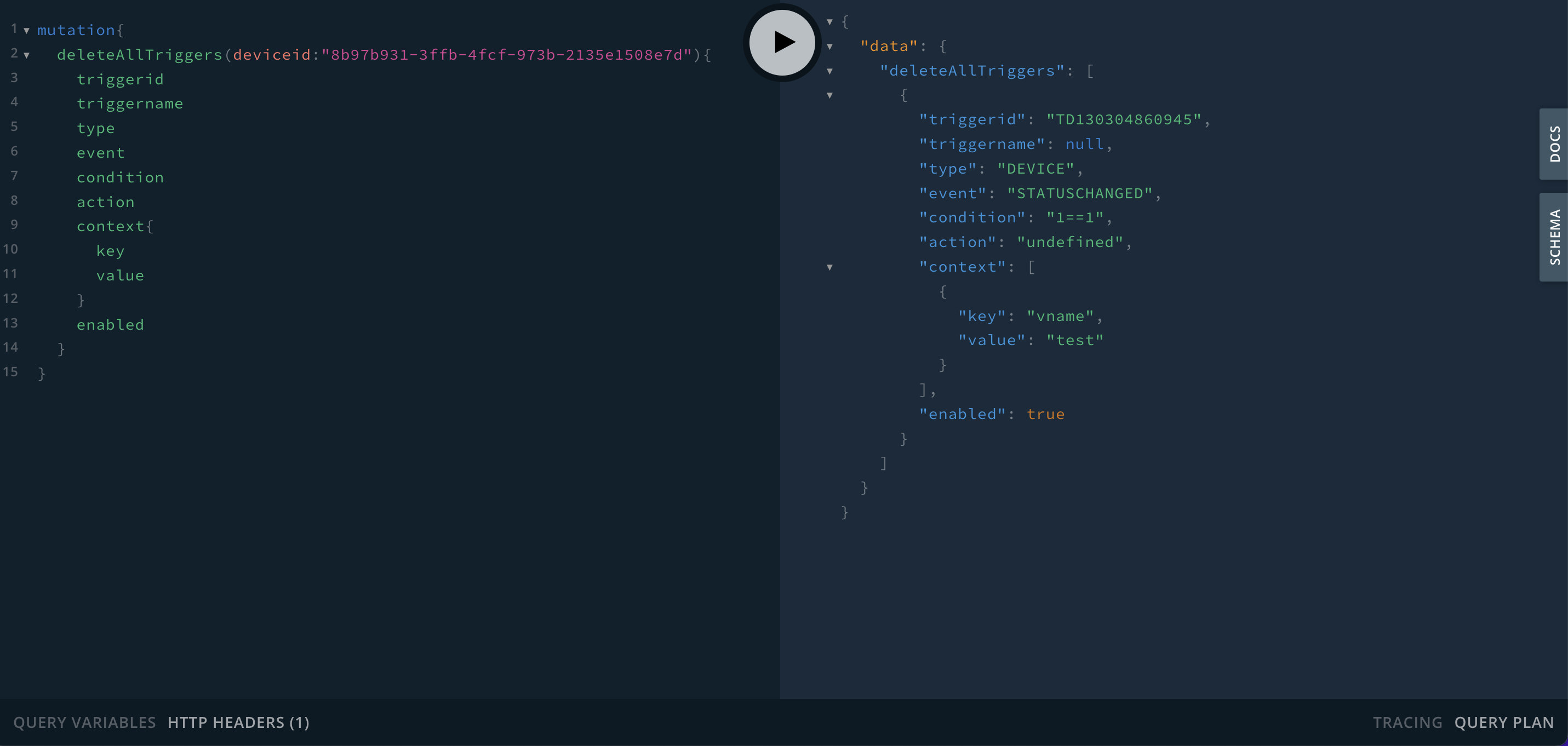
DeleteDevice¶
To delete a device by ID, the details are as follows:
deleteDevice ( deviceid ):
Arguments
deviceid
: String is the Device ID (required)
Query Variables
Authorization
: User Token
Response Type : Device
Response is a deleted Object Type Device consisting of
alias
: String is the name of the Device.
createdtime
: Timestamp is the date and time the Device was created.
credential
: Credential is data for verifying identity. Object Type credential consists of:
secret
: String is the Device's secret code for Authentication.
description
: String is a Device description.
deviceid
: String is the Device ID.
devicesecret
: String is the secret code of the Device.
groupid
: String is the ID of the Group that the Device is under.
groupname
: String is the name of the Group that the Device is under.
projectid
: String is the Project ID that the Device is under.
status
: Int is Device’s connection status to the platform (0
offline,1
online)
tag
: String is a Device tag in single value format.
hashtag
: [String] is the Device tag in multi-value format.
tags
: Json is a Device tag in Key Value format.
enabled
: Boolean is the Device activation status (true
enabled [default],false
disabled).
banned
: Boolean is the status of being suspended from the system (true
suspended,false
not suspended)
An example is shown in the following figure:
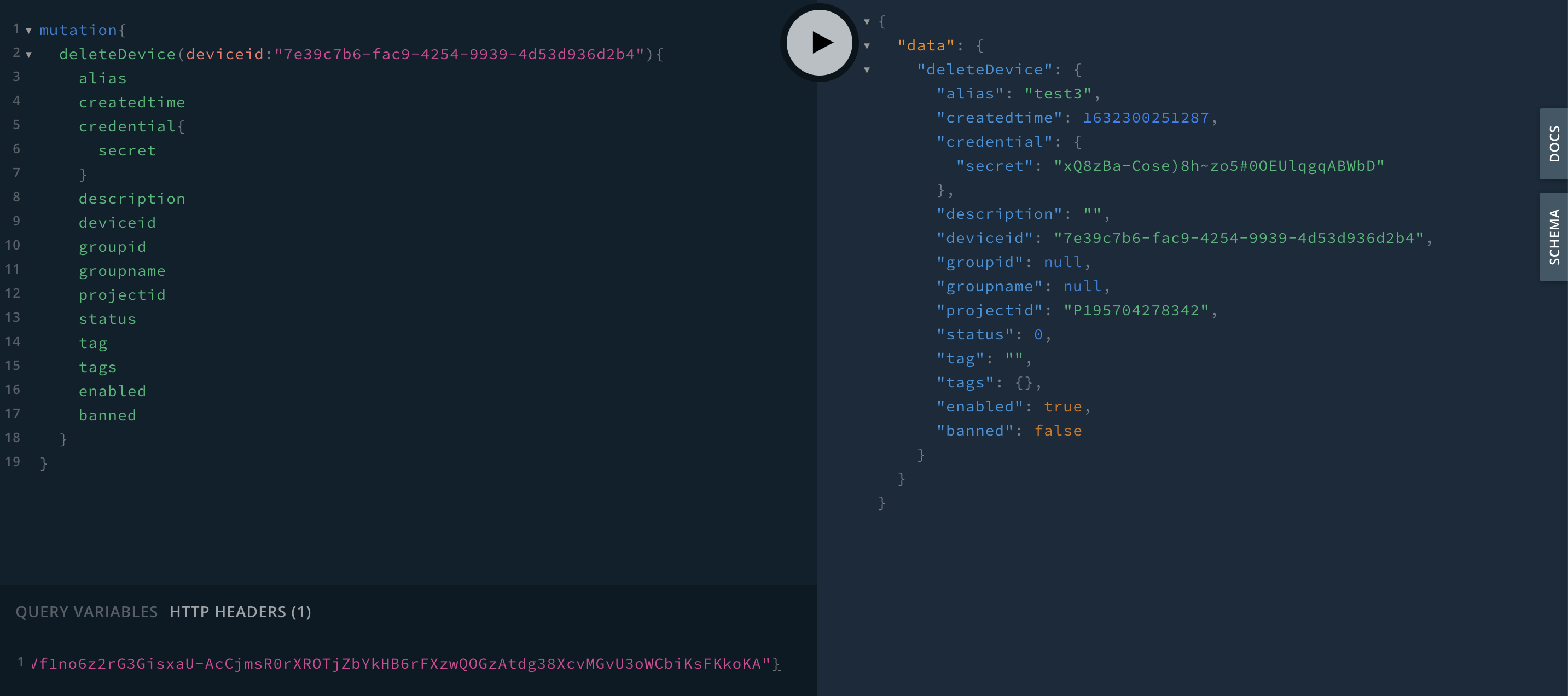
DeleteFeedData¶
To delete some data from the Time Series Data Store of that Device, such as data from invalid ranges, details are as follows:
deleteFeedData ( feedid, attributes, starttime, endtime ):
Arguments
feedid
: String is the Feed ID which has the same value asdeviceid
(required).
attributes
: [String] is the name of the field to be deleted (if not specified, all fields will be deleted).
starttime
: Timestamp is the start time period for which you want to delete the data (not specified means delete all).
endtime
: Timestamp is the end time period for which you want to delete the data (not specified means delete all).
Query Variables
Authorization
: User Token
Response Type : FeedData
The response is Object Type FeedData consisting of:
feedid
: String is the Feed ID.
data
: JSON is the data in each field.
An example is shown in the following figure:
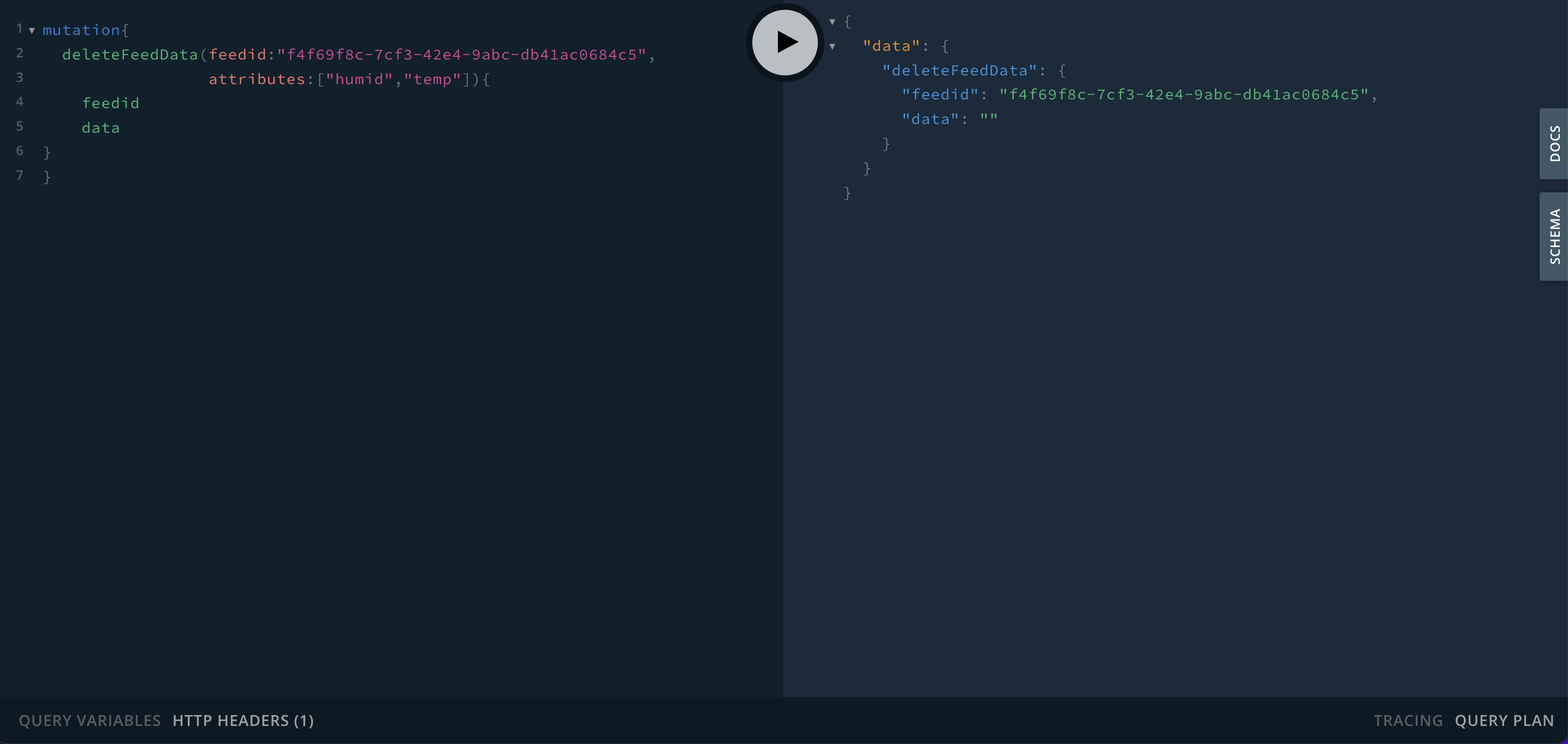
DeleteGroup¶
To delete a Group by ID, the details are as follows:
deleteGroup ( groupid ):
Arguments
groupid
: String is the Group ID (required).
Query Variables
Authorization
: User Token
Response Type : Group
The reply is a deleted Object Type Group consisting of:
groupid
: String is the Group ID.
groupname
: String is the Group Name.
projectid
: String is the Project ID.
createdtime
: Timestamp is the date and time the Group was created.
description
: String is a description of the Group.
tag
: String is a single-valued Group tag.
hashtag
: [String] is a multi-valued Group tag
tags
: Json is a Group tag in Key Value format.
An example is shown in the following figure:
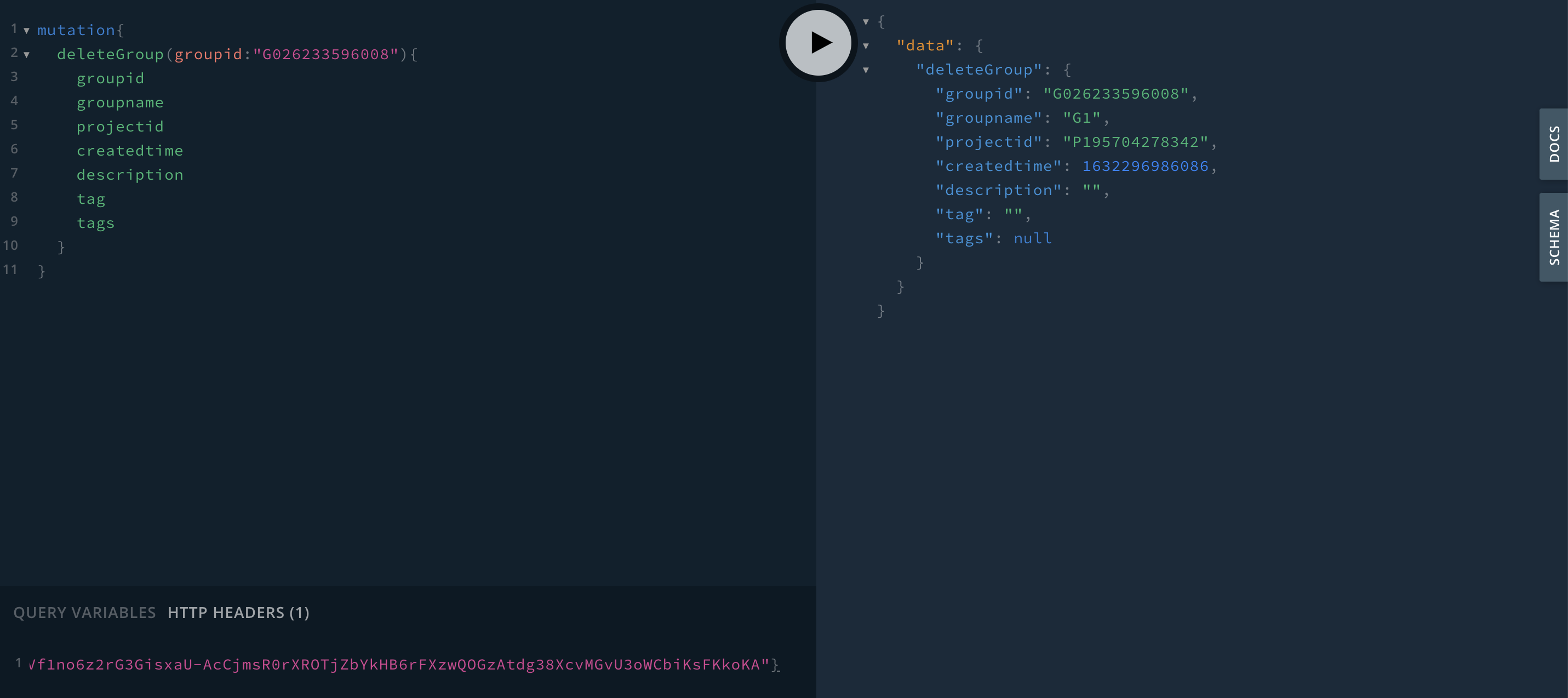
DeleteHook¶
To delete a Hook with ID, the details are as follows:
deleteHook ( projectid, hookid ):
Arguments
projectid
: String is the Project ID (required)
hookid
: String is the Hook ID (required).
Query Variables
Authorization
: User Token
Response Type : Hook
The response is a deleted Object Type Hook, consisting of:
projectid
: String is the Project ID.
name
: String is the name of the Hook.
hookid
: String is the ID of Hook.
description
: String is a description of Hook.
type
: String is a type of Hook.
enabled
: Boolean is the state of the Hook (true
enabled,false
disabled).
param
: JSON is the various parameters set in the Hook.
An example is shown in the following figure:
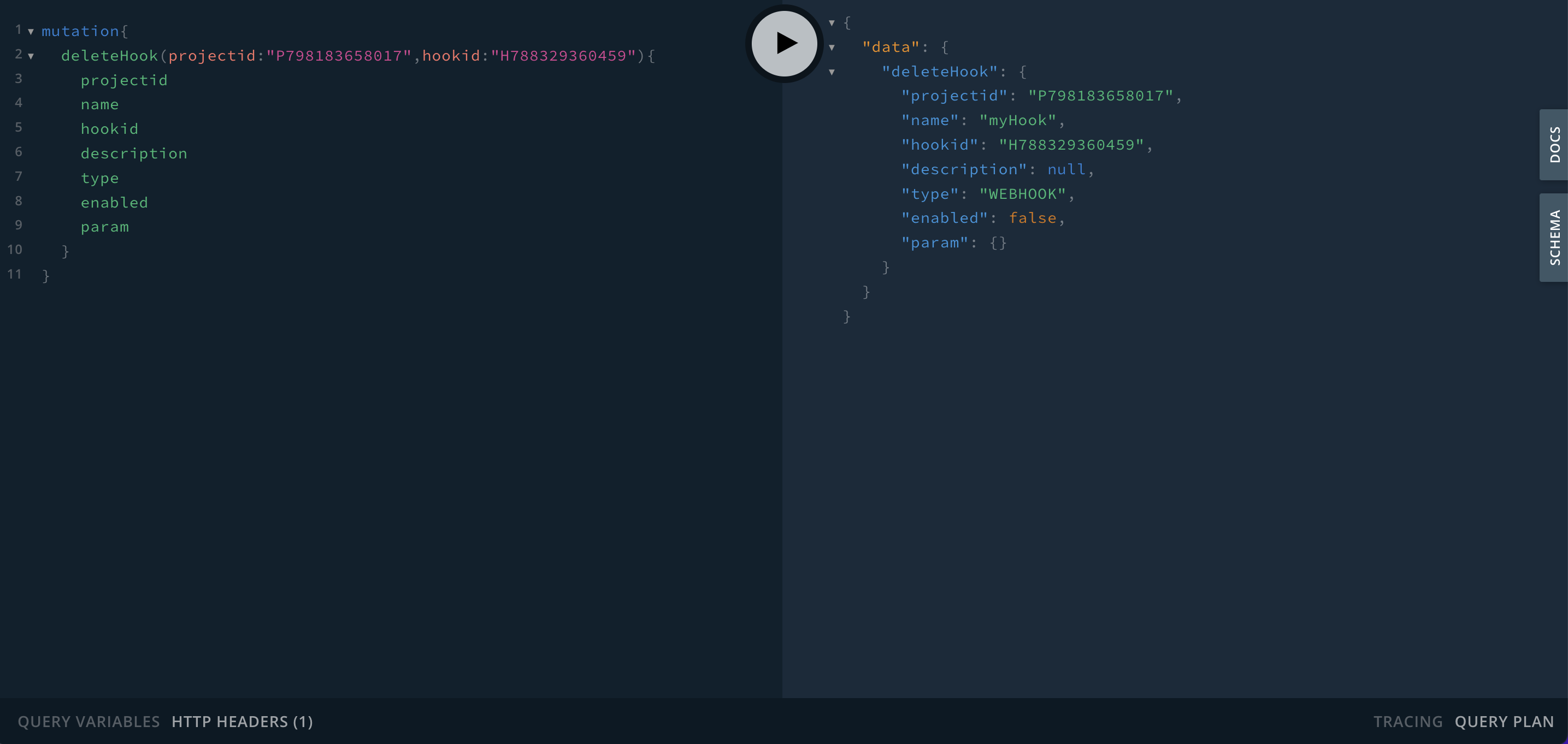
DeleteMembership¶
To delete Membership in each Project, details are as follows:
deleteMembership ( projectid, username ):
Arguments
projectid
: String is the Project ID (required)
username
: String is the username of the user who is a member and wants to be deleted (required).
Query Variables
Authorization
: User Token
Response Type : Membership
Response is a deleted Object Type Membership consisting of
createdtime
: Timestamp is the date and time the Membership was created.
level
: Int is the membership level.
projectid
: String is the Project ID that the Membership is under.
userid
: Striing is the User ID.
username
: String is the username of the member user.
An example is shown in the following figure:
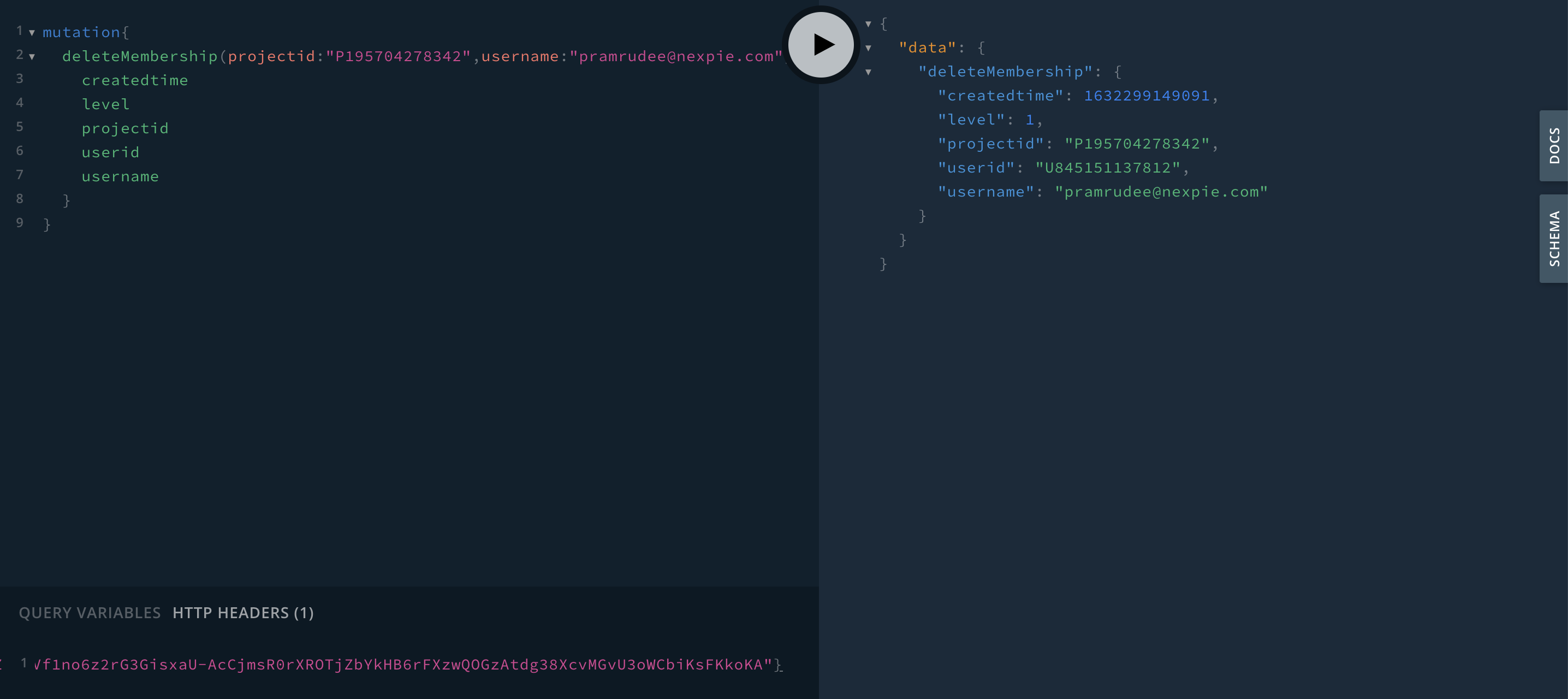
DeleteProject¶
To delete a Project with ID, the details are as follows:
deleteProject ( projectid ):
Arguments
projectid
: String is the Project ID (required)
Query Variables
Authorization
: User Token
Response Type : Project
Response is a deleted Object Type Project consisting of
projectname
: String is the name of the Project.
projectid
: String is the Project ID.
createdtime
: Timestamp is the date and time the project was created.
description
: String is a project description.
userlevel
: String is the user's project access level.
tags
: Json is a project tag in Key Value format.
tag
: String is a single-valued Project tag.
hashtag
: [String] is a multi-valued Project tag.
numberdevice
: Int is the number of devices under the project.
numbergroup
: Int is the number of Groups under the Project.
numberdeviceonline
: Int is the number of devices currently connected to the Platform under the Project.
numberdeviceoffline
: Int is number of Devices currently not connected to the Platform under the Project.
quota
: Quota is the status of each service with available quota, which includes:
apicall
: Boolean is a REST API service (true
available quota,false
unavailable or no quota)
connection
: Boolean คือ บริการเชื่อมต่อ Platform ของ Device (true
available quota,false
unavailable or no quota).
message
: Boolean is a Real Time Message service via MQTT Protocol (true
available quota,false
unavailable or no quota).
shadowops
: Boolean is a Shadow read/write service (true
available quota,false
unavailable or no quota)
store
: Boolean is a service that stores data in Time Series Data (true
available quota,false
unavailable or no quota)
trigger
: Boolean is Trigger & Action (Notification) service (true
available quota,false
unavailable or no quota)
datasource
: Boolean is the sum of the Byte size of the data that has been used by theapicall
service (true
available quota,false
unavailable or no quota)
An example is shown in the following figure:
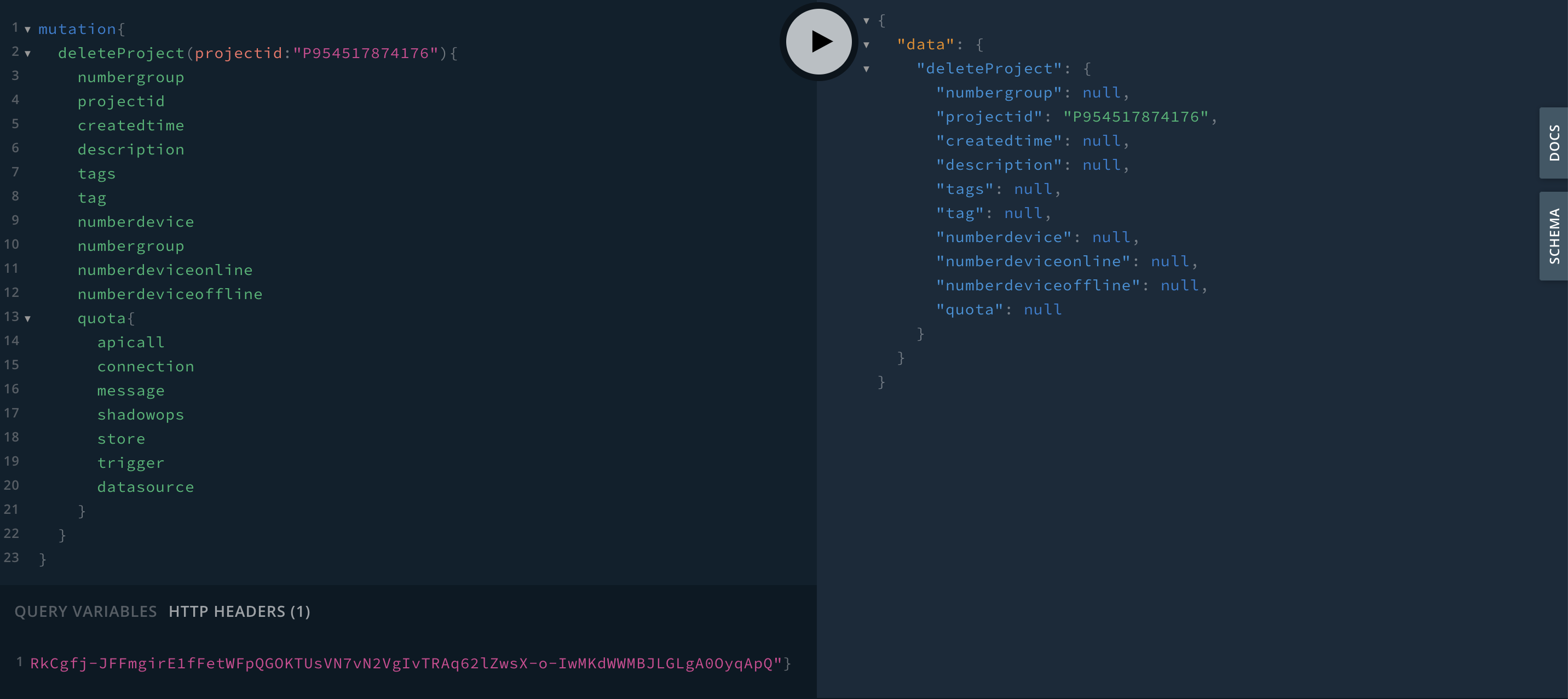
DeleteTrigger¶
To delete individual Triggers, the details are as follows:
deleteTrigger ( deviceid, triggerid ):
Arguments
deviceid
: String is the Device ID that is set to trigger (required)
triggerid
: String is the Trigger ID (required)
Query Variables
Authorization
: User Token
Response Type : Trigger
The response is an Object Type Trigger that was deleted, consisting of:
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String is the event that triggers (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
).
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
An example is shown in the following figure:
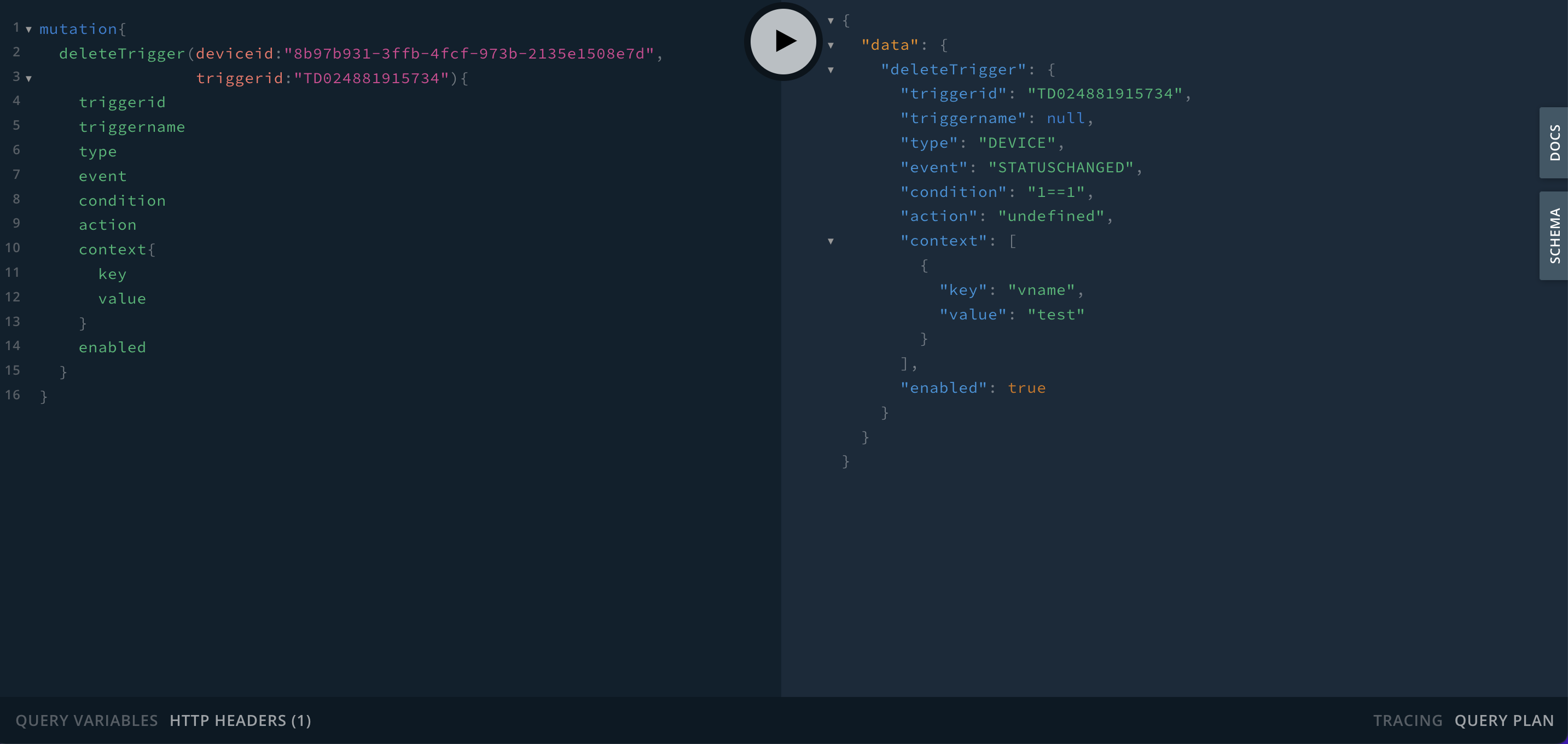
DisableAllTriggers¶
To disable all Triggers under that Device, details are as follows:
disableAllTriggers ( deviceid ):
Arguments
deviceid
: String is the Device ID that is set to trigger (required)
Query Variables
Authorization
: User Token
Response Type : [Trigger]
The response is an Array of disabled Object Type Triggers, consisting of:
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String is the event that triggers (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
).
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
An example is shown in the following figure:
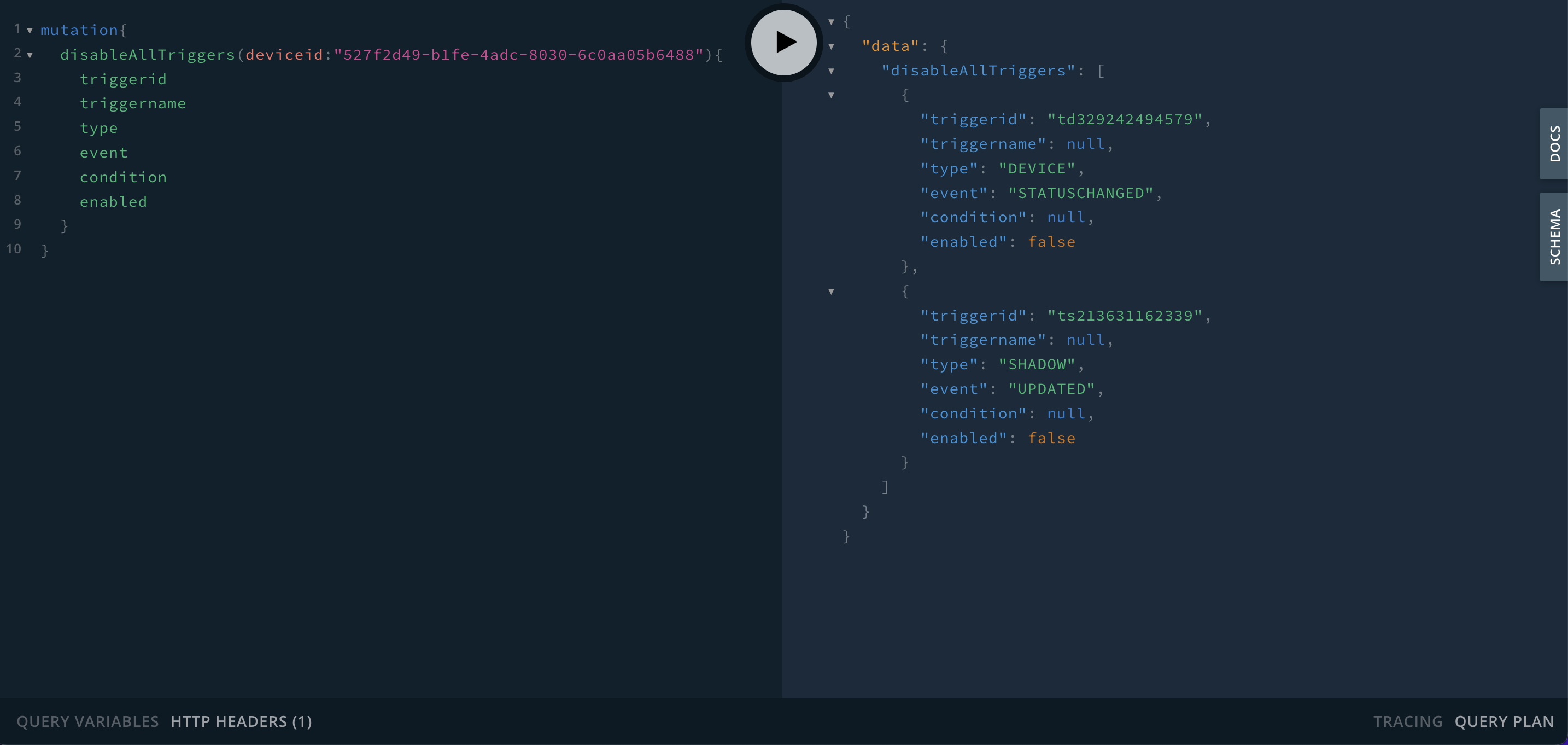
DisableDevice¶
For each device disable set by DeviceID (set enabled
is false
) details as follows:
disableDevice ( deviceid ):
Arguments
deviceid
: String is the ID of the Device to be disabled (required)
Query Variables
Authorization
: User Token
Response Type : Trigger
Response is a deleted Object Type Device consisting of
alias
: String is the name of the Device.
createdtime
: Timestamp is the date and time the Device was created.
credential
: Credential is data for verifying identity. Object Type credential consists of:
secret
: String is the Device's secret code for Authentication.
description
: String is a Device description.
deviceid
: String is the Device ID.
devicesecret
: String is the secret code of the Device.
groupid
: String is the ID of the Group that the Device is under.
groupname
: String is the name of the Group that the Device is under.
projectid
: String is the Project ID that the Device is under.
status
: Int is the Device's Platform connection status (0
offline,1
online).
tag
: String is a single-valued Device tag.
hashtag
: [String] is a multi-valued Device tag.
tags
: JSON is a Device tag in Key Value format.
enabled
: Boolean is the Device activation status (true
enabled [default],false
disabled).
banned
: Boolean is the status of being suspended from the system (true
suspended,false
not suspended)
An example is shown in the following figure:
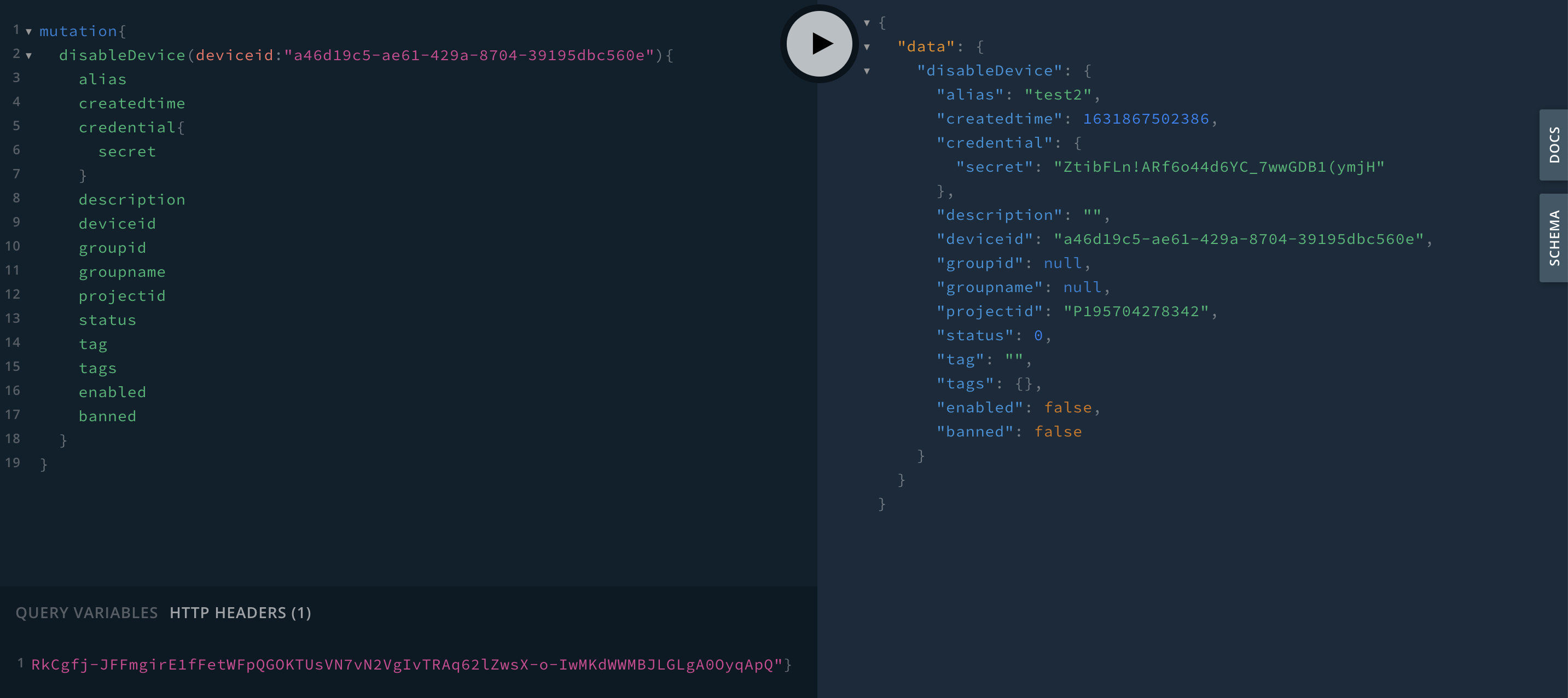
DisableTrigger¶
To disable individual Triggers, the details are as follows:
disableTrigger ( deviceid, triggerid ):
Arguments
deviceid
: String is the Device ID that is set to trigger (required)
triggerid
: String is the Trigger code (required)
Query Variables
Authorization
: User Token
Response Type : Trigger
The response is a disabled Object Type Trigger, consisting of:
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: String คือ is of Trigger type (DEVICE
,SHADOW
)
event
: String is the event that triggers (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
).
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
An example is shown in the following figure:
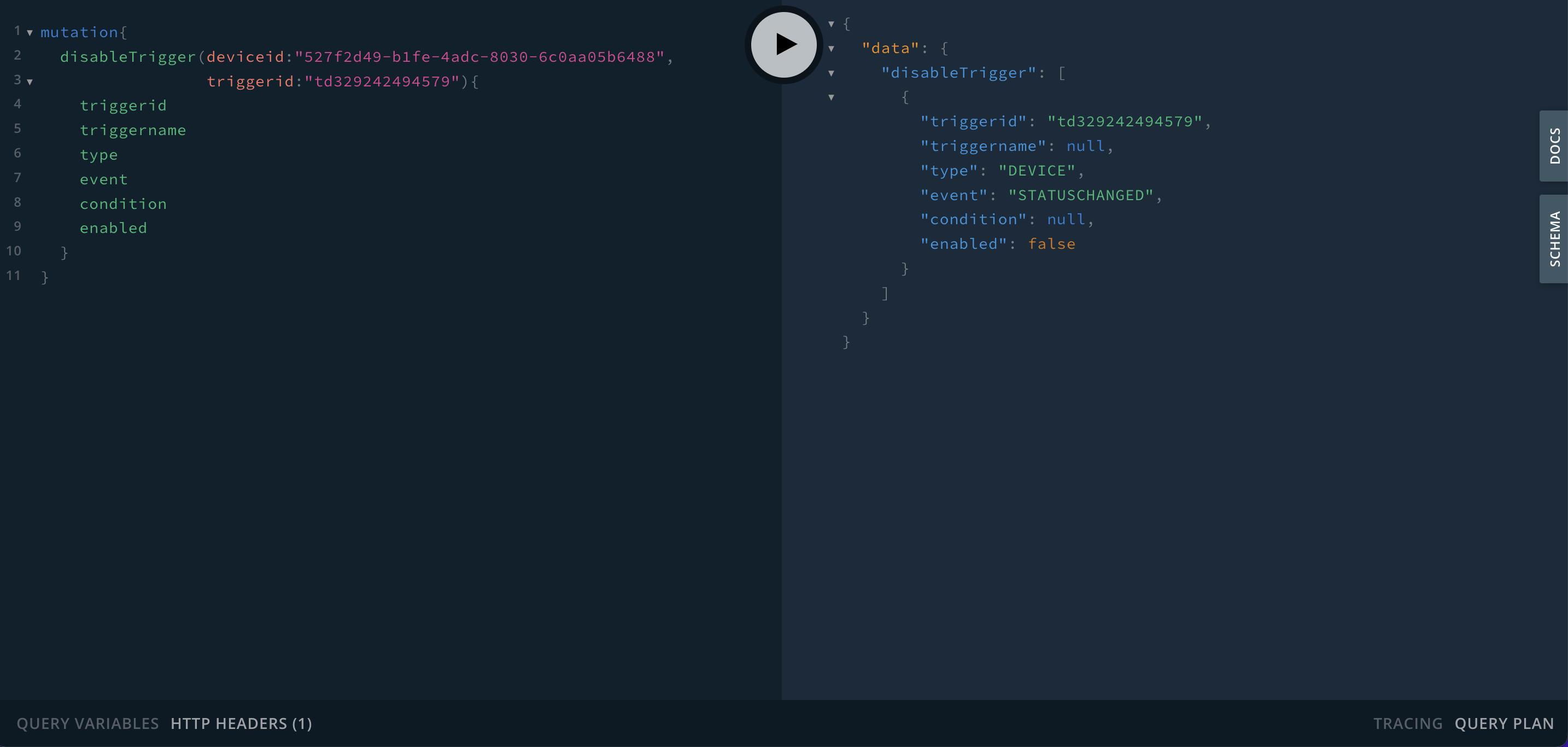
EnableAllTriggers¶
To enable all Triggers under that Device, the details are as follows:
disableAllTriggers ( deviceid ):
Arguments
deviceid
: String is the Device ID that is set to trigger (required)
Query Variables
Authorization
: User Token
Response Type : [Trigger]
The response is an Array of enabled Object Type Triggers, consisting of:
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String is the event that triggers (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
).
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
An example is shown in the following figure:
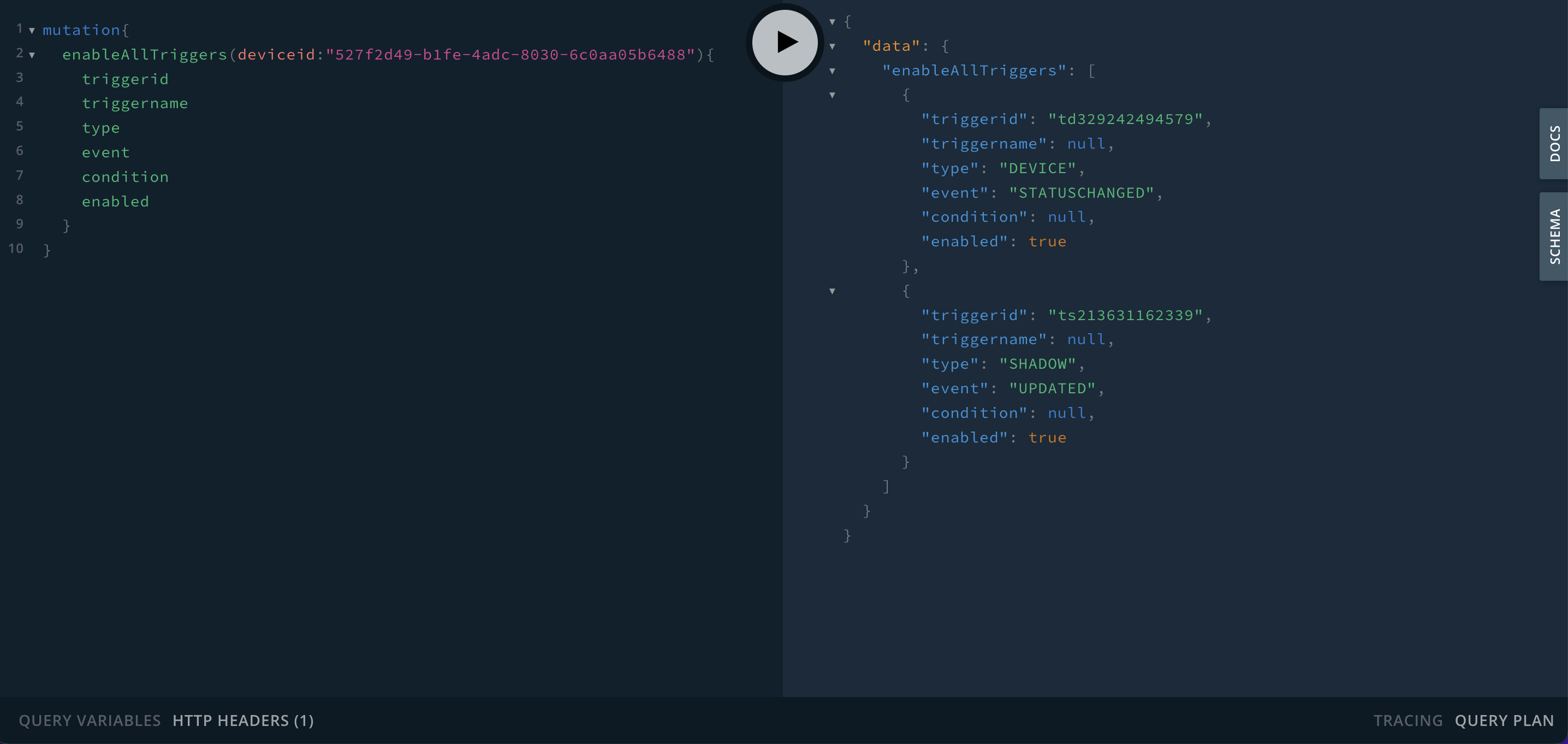
EnableDevice¶
For each enabled device set with DeviceID (set enabled
is true
) details are as follows:
enableDevice ( deviceid ):
Arguments
deviceid
: String is the code of the Device to be enabled (required)
Query Variables
Authorization
: User Token
Response Type : Trigger
Response is a deleted Object Type Device consisting of
alias
: String is the name of the Device.
createdtime
: Timestamp is the date and time the Device was created.
credential
: Credential is data for verifying identity. Object Type credential consists of:
secret
: String is the Device's secret code for Authentication.
description
: String is a Device description.
deviceid
: String is the Device ID.
devicesecret
: String is the secret code of the Device.
groupid
: String is the code of the Group that the Device is under.
groupname
: String is the name of the Group that the Device is under.
projectid
: String is the Project ID that the Device is under.
status
: Int is the Device's Platform connection status (0
offline,1
online).
tag
: String is a single-valued Device tag.
hashtag
: [String] is a multi-valued Device tag.
tags
: JSON is a Device tag in Key Value format.
enabled
: Boolean is the Device activation status (true
enabled [default],false
disabled).
banned
: Boolean is the status of being suspended from the system (true
suspended,false
not suspended)
An example is shown in the following figure:
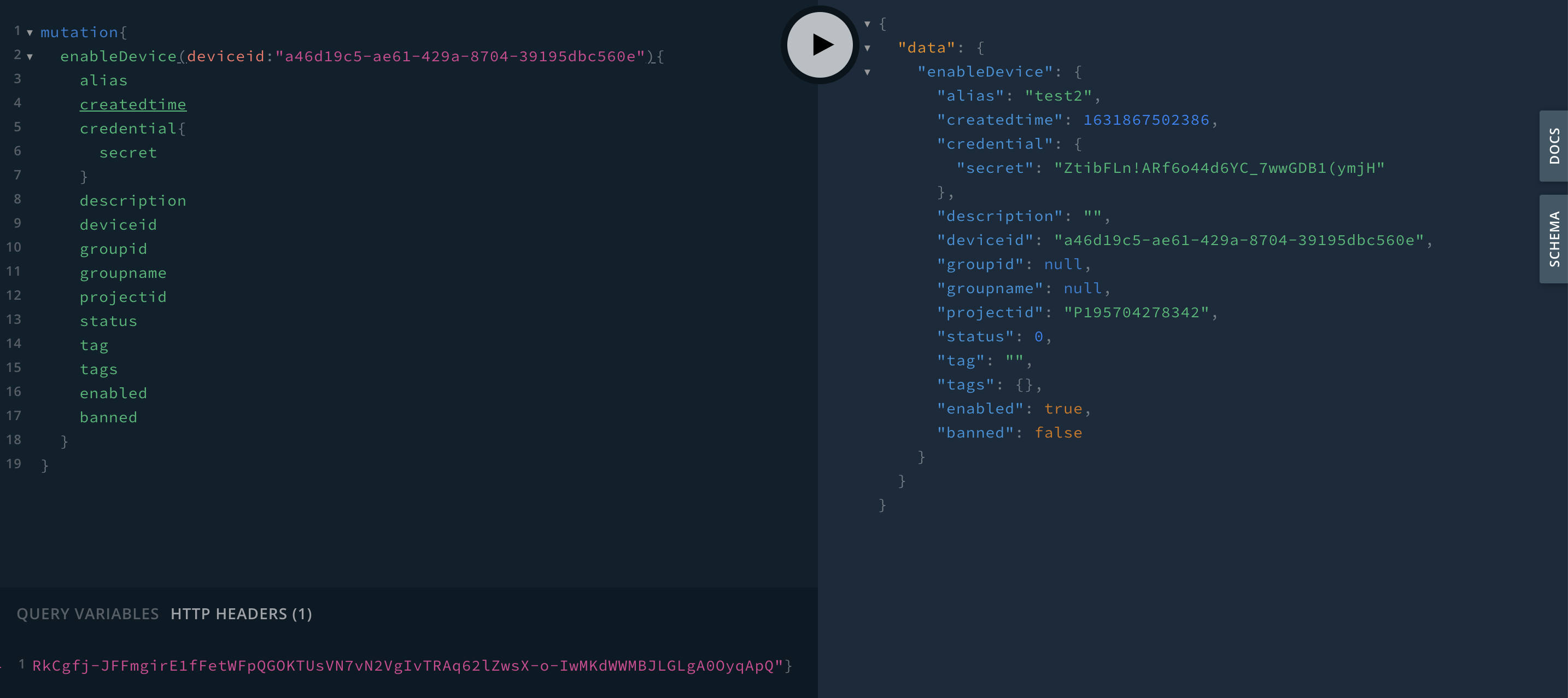
EnableTrigger¶
To enable individual triggers, the details are as follows:
enableTrigger ( deviceid, triggerid ):
Arguments
deviceid
: String is the Device ID that is set to trigger (required)
triggerid
: String is the Trigger code (required)
Query Variables
Authorization
: User Token
Response Type : Trigger
The response is an Object Type Trigger that is enabled, including:
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String is the event that triggers (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
).
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
An example is shown in the following figure:
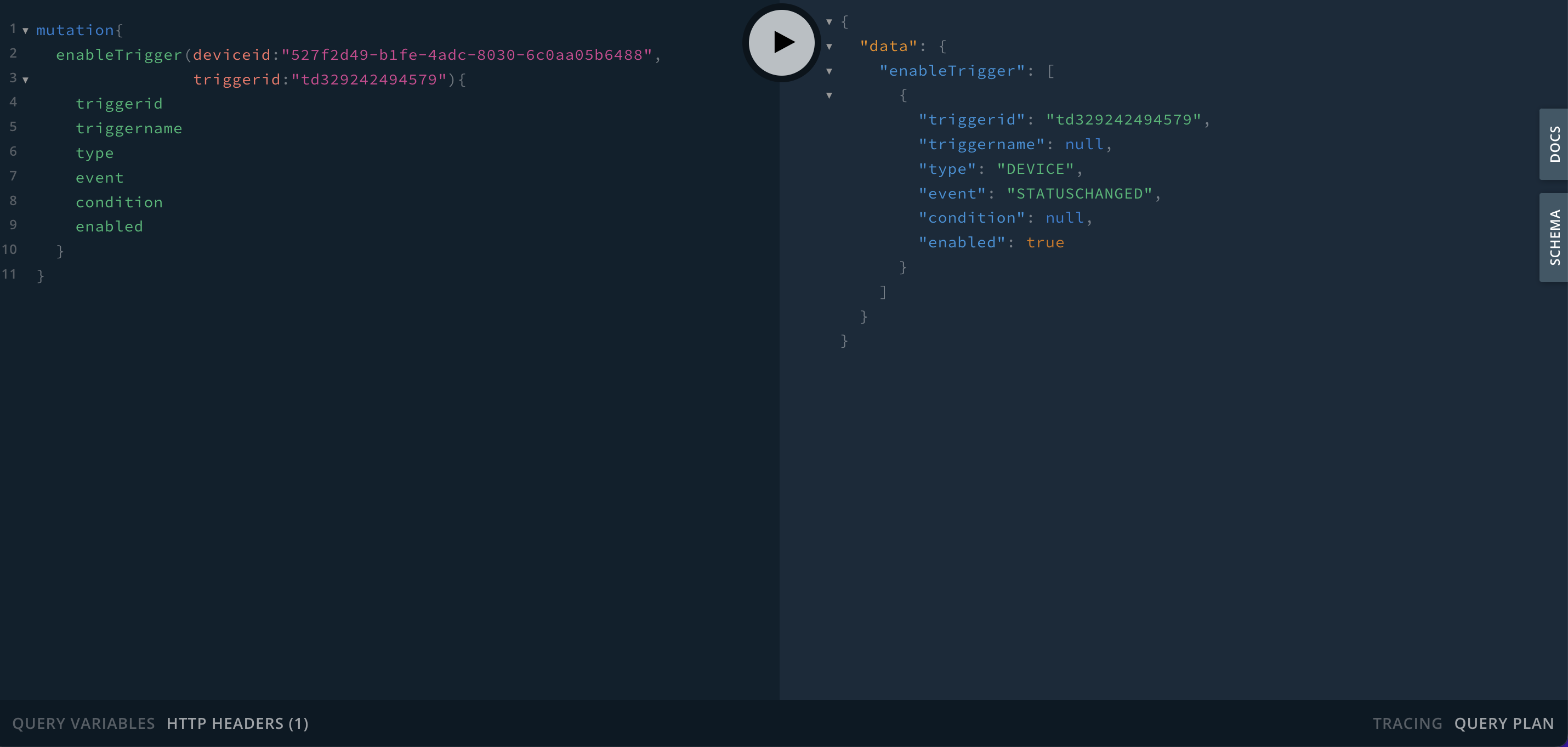
GroupDevice¶
To set a Group for Devices, the details are as follows:
groupDevice ( deviceid, groupid ):
Arguments
deviceid
: [String] is an Array of Device IDs to be grouped (required).
groupid
: String is the Group ID to be assigned to Devices (required).
Query Variables
Authorization
: User Token
Response Type : [Device]
The response is an Array of the specified Device Type Objects. The Group contains:
alias
: String is the name of the Device.
createdtime
: Timestamp is the date and time the Device was created.
credential
: Credential is data for verifying identity. Object Type credential consists of:
secret
: String is the Device's secret code for Authentication.
description
: String is a Device description.
deviceid
: String is the Device ID.
devicesecret
: String is the secret code of the Device.
groupid
: String is the ID of the Group that the Device is under.
groupname
: String is the name of the Group that the Device is under.
projectid
: String is the Project ID that the Device is under.
status
: Int is the Device's Platform connection status (0
offline,1
online).
tag
: String is a single-valued Device tag.
hashtag
: [String] is a multi-valued Device tag.
tags
: Json is a Device tag in Key Value format.
enabled
: Boolean is the Device activation status (true
enabled [default],false
disabled).
banned
: Boolean is the status of being suspended from the system (true
suspended,false
not suspended)
An example is shown in the following figure:
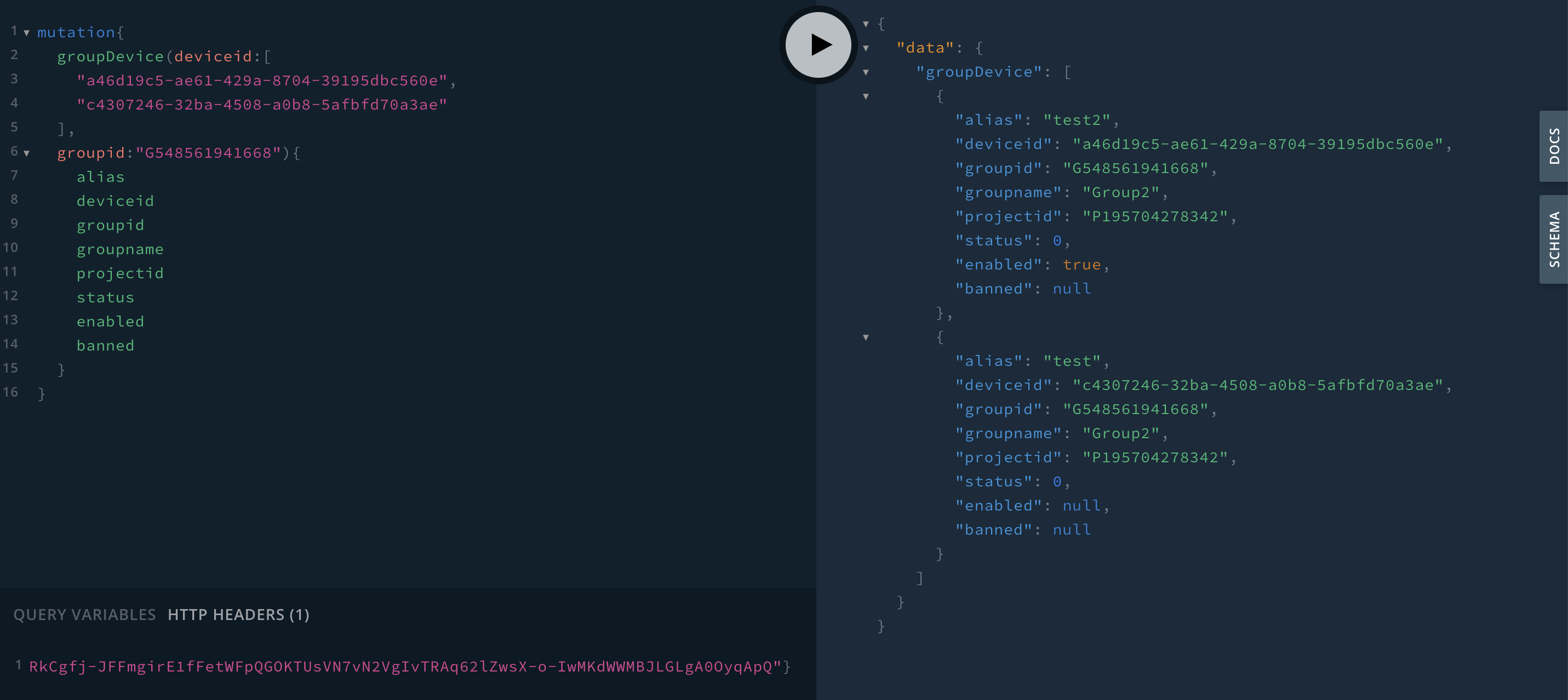
LeaveProject¶
For resigning from membership in each Project that you are entitled to, the details are as follows:
leaveProject ( projectid ):
Arguments
projectid
: String is the ID of the Project you want to remove from membership (required)
Query Variables
Authorization
: User Token
Response Type : Membership
Response is a deleted Object Type Membership consisting of
createdtime
: Timestamp is the date and time of membership.
level
: UserLevel is the level of membership privileges. There are 5 levels in total (for details of privileges, see Member)
5
(Owner)
4
(Master)
3
(Editor)
2
(Viewer)
1
(Guest)
projectid
: String is the ID of the project that is leaving the membership.
userid
: String is the member user ID.
username
: String is the username of the member user.
An example is shown in the following figure:
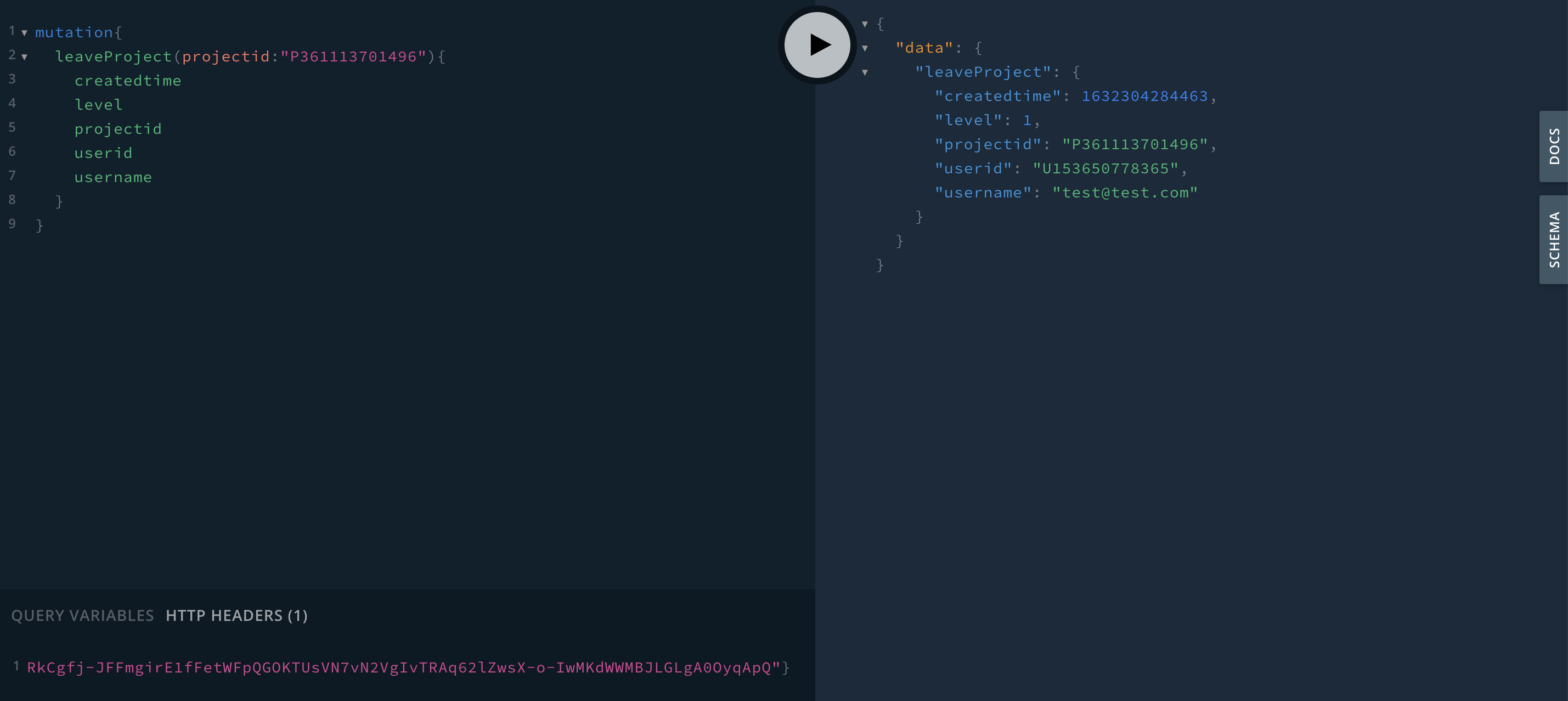
MoveDeviceToProject¶
To move a device to the desired project, the details are as follows:
moveDeviceToProject ( deviceid, devicesecret, projectid ):
Arguments
deviceid
: String is the Device ID to move the Project to. (required)
devicesecret
: String is the secret code of the Device to move the Project to.(required)
projectid
: String is the Project ID that the Device must be moved to (required)
Query Variables
Authorization
: User Token
Response Type : DeviceWithToken
The response is a deleted Object Type DeviceWithToken consisting of:
alias
: String is the name of the Device.
createdtime
: Timestamp is the date and time the Device was created.
credential
: Credential is data for verifying identity. Object Type credential consists of:
secret
: String is the Device's secret code for Authentication.
description
: String is a Device description.
deviceid
: String is the Device ID.
devicetoken
: [String] is a list of Device Tokens.
devicesecret
: String is the secret code of the Device.
groupid
: String is the ID of the Group that the Device is under.
groupname
: String is the name of the Group that the Device is under.
projectid
: String is the Project ID that the Device is under.
status
: Int is the Device's Platform connection status (0
offline,1
online).
tag
: String is a single-valued Device tag.
hashtag
: [String] is a multi-valued Device tag.
tags
: JSON คืis a Device tag in Key Value format.
enabled
: Boolean is the Device activation status (true
enabled [default],false
disabled).
banned
: Boolean is the status of being suspended from the system (true
suspended,false
not suspended)
token
: Token is an Object Type of Token data. It consists of:
tokencode
: String is the code of the Token.
iat
: String is the date and time the token was issued (issued at).
nbf
: String is the date when the token will be active (not before).
exp
: String is the expiration time of the Token.
expireIn
: String is the age of the Token.
An example is shown in the following figure:
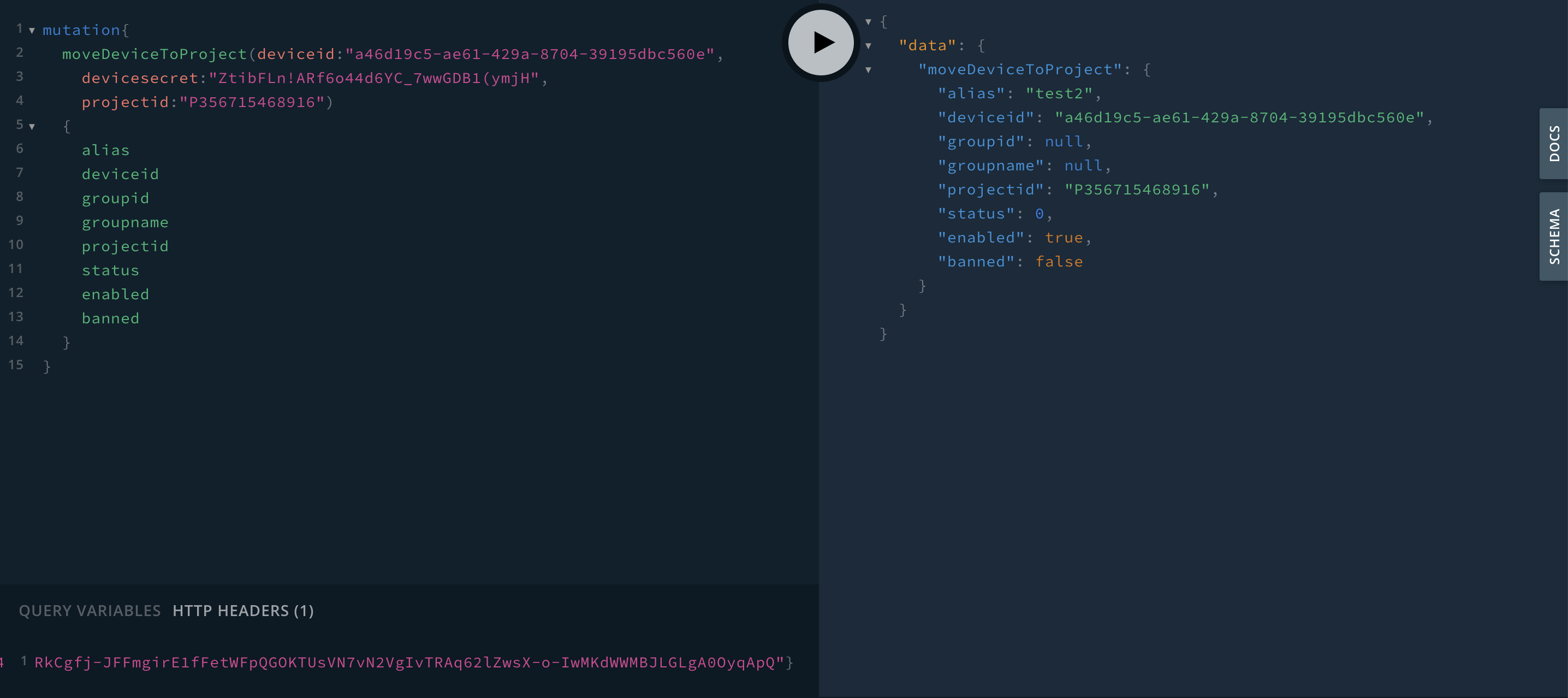
PublishMessageToDevice¶
For publishing specific data to each device, suitable for use with applications developed to communicate with devices. The User Token used for Authorization must belong to the owner of that device. Details are as follows:
publishMessageToDevice ( deviceid, topic, payload ):
Arguments
deviceid
: String is the ID of the Device to which you want to send the data (required)
topic
: String is the Topic to which you want to publish the information, without specifying @msg (required).
payload
: String คือ ข้อมูลหรือข้อความที่ต้องการส่ง
Query Variables
Authorization
: User Token
Response Type : Result
The response is an Object Type Result consisting of:
code
: Int is the Response Code. If it is 200, it means the operation was successful.
text
: String is the response text.
An example is shown in the following figure:
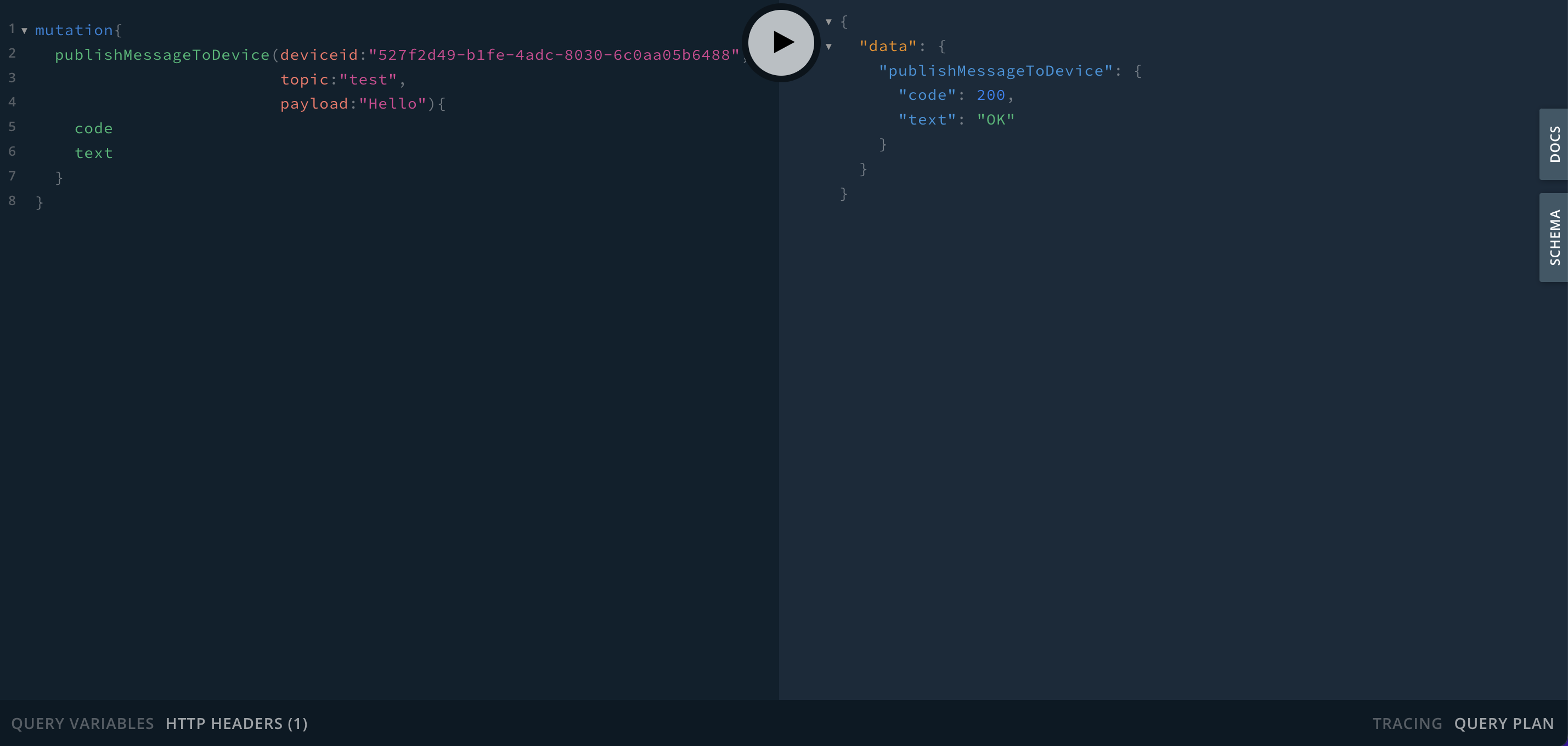
Note
PublishMessageToDevice
Publishing data in this function, Topic setting does not need to be @msg
preceded.
Subscribing to data coming from the PublishMessageToDevice function will have a Topic prefix such @private
as:
publishMessageToDevice (deviceid: "c55941ce-0f4a-4e57-xxxx-4759af4f872c", topic: "home/door", payload: "open" )
The topic setting when subscribing is as follows: @private/home/door
PublishMessageToGroup¶
For publishing data to devices under that group. Suitable for use with applications developed to communicate with devices. The User Token used for Authorization must belong to the owner of that device. Details are as follows:
publishMessageToGroup ( groupid, topic, payload ):
Arguments
groupid
: String is the ID of the Group to which you want to send the data (required).
topic
: String is the Topic to which you want to publish the information, without specifying @msg (required).
payload
: String is the data or message to be sent.
Query Variables
Authorization
: User Token
Response Type : Result
The response is an Object Type Result consisting of:
code
: Int is the Response Code. If it is 200, it means the operation was successful.
text
: String is the response text.
An example is shown in the following figure:
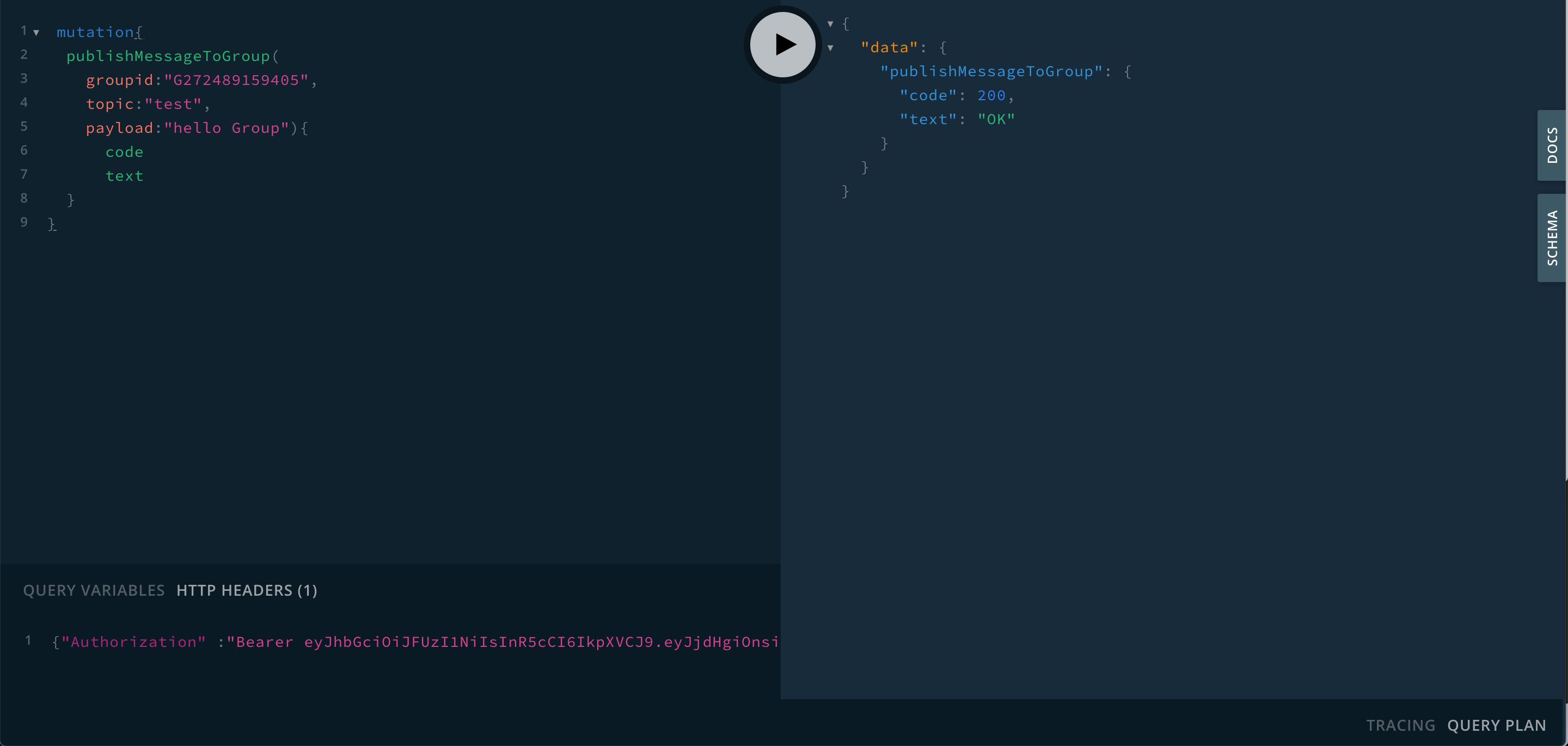
Note
PublishMessageToGroup
Publishing data in this function, Topic setting does not need to be @msg
preceded.
Subscribing to data coming from the PublishMessageToGroup function will have a Topic prefix such @msg
as:
publishMessageToGroup (groupid: "G272489159405", topic: "home/rooms", payload: "open" )
The topic setting when subscribing is as follows: @msg/home/rooms
ResyncDeviceStatus¶
To sync the device platform connection status data. In case the device status is displayed on the UI and the status does not update automatically, call this function to sync the data. Details are as follows:
resyncDeviceStatus ( deviceid ):
Arguments
deviceid
: String is the Device ID to synchronize data (required)
Query Variables
Authorization
: User Token
Response Type : Result
The response is an Object Type Result consisting of:
code
: Int is the Response Code. If it is 200, it means the operation was successful.
text
: String is the response text.
An example is shown in the following figure:
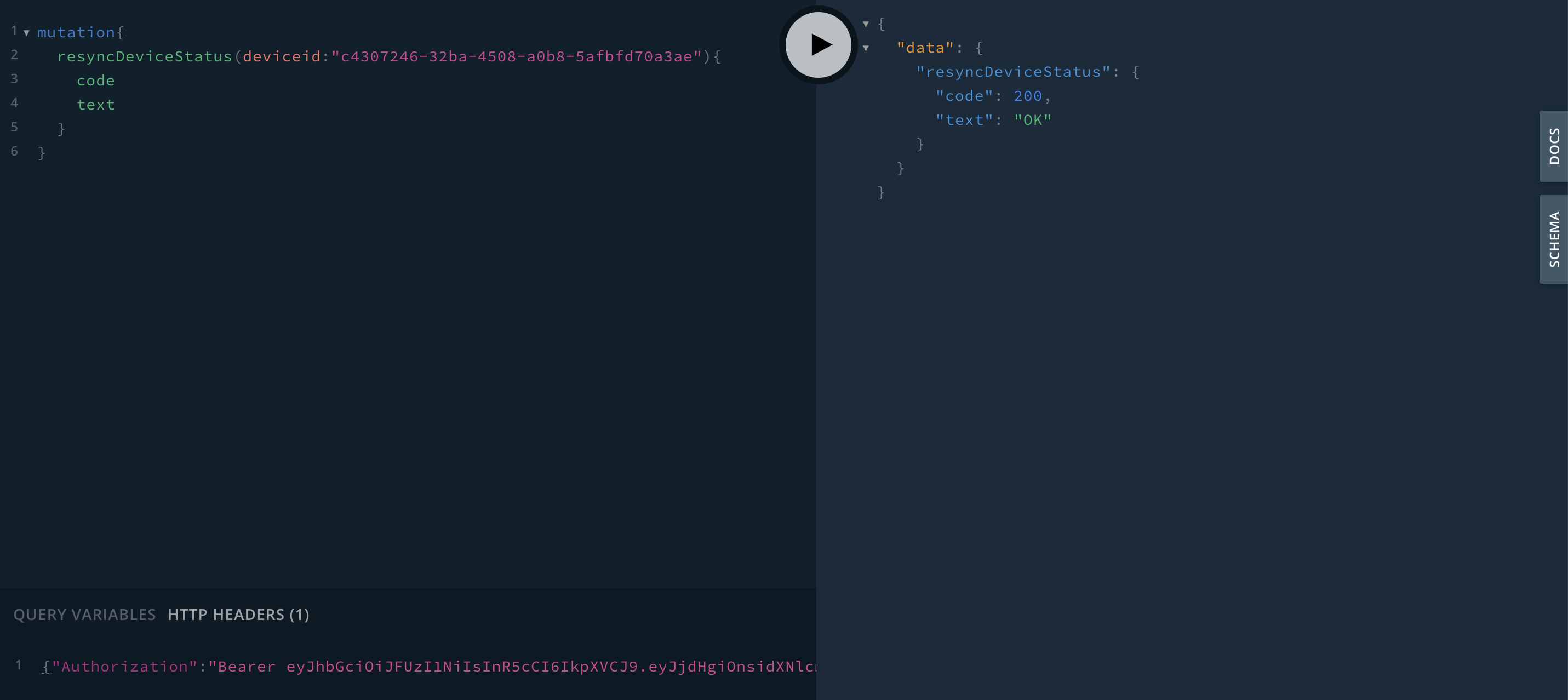
RevokeDeviceToken¶
To cancel the Token rights of the Device, the details are as follows:
revokeDeviceToken ( tokencode ):
Arguments
tokencode
: String is the code of the Token that you want to revoke the rights to (required)
Query Variables
Authorization
: User Token
Response Type : DeviceToken
The response is an object of type DeviceToken that has been deprecated, consisting of:
deviceid
: String is the Device ID.
tokencode
: String is the code of the Token.
iat
: String is the date and time the token was issued (issued at).
nbf
: String is the date when the token will be active (not before).
exp
: String is the expiration time of the Token.
expireIn
: String is the age of the Token.
An example is shown in the following figure:
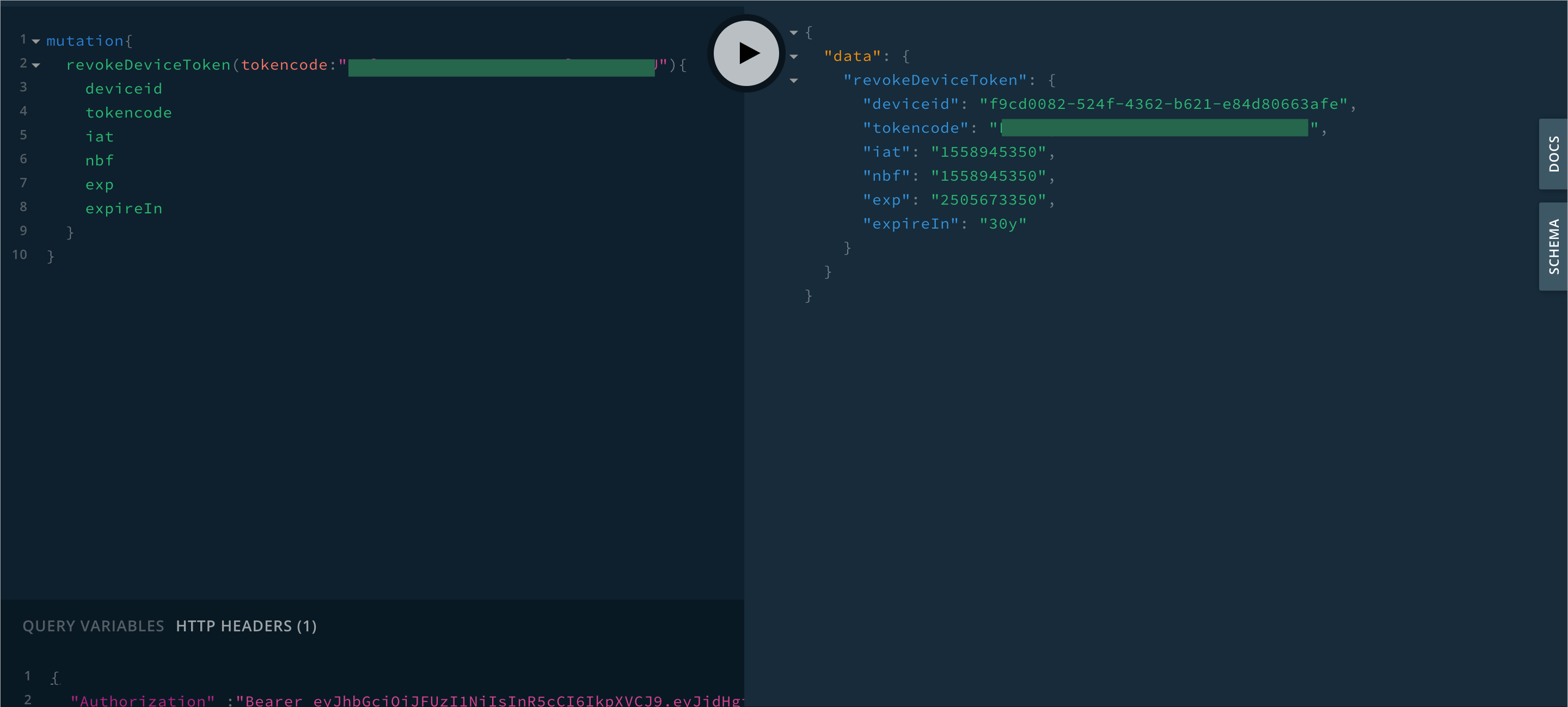
SaveTrigger¶
To update multiple Trigger data at once, the details are as follows:
saveTrigger ( deviceid, triggers ):
Arguments
deviceid
: String is the Device ID that is set to trigger (required)
trigger
: [TriggerInput] is an Array of all Object Type Triggers (all values must be specified), consisting of:
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String is the event that triggers (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
).
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
Query Variables
Authorization
: User Token
Response Type : [Trigger]
The response is an Array of enabled Object Type Triggers, consisting of:
triggerid
: String is the Trigger ID.
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String is the event that triggers (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATEDpaired
with Trigger typeSHADOW
).
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
An example is shown in the following figure:
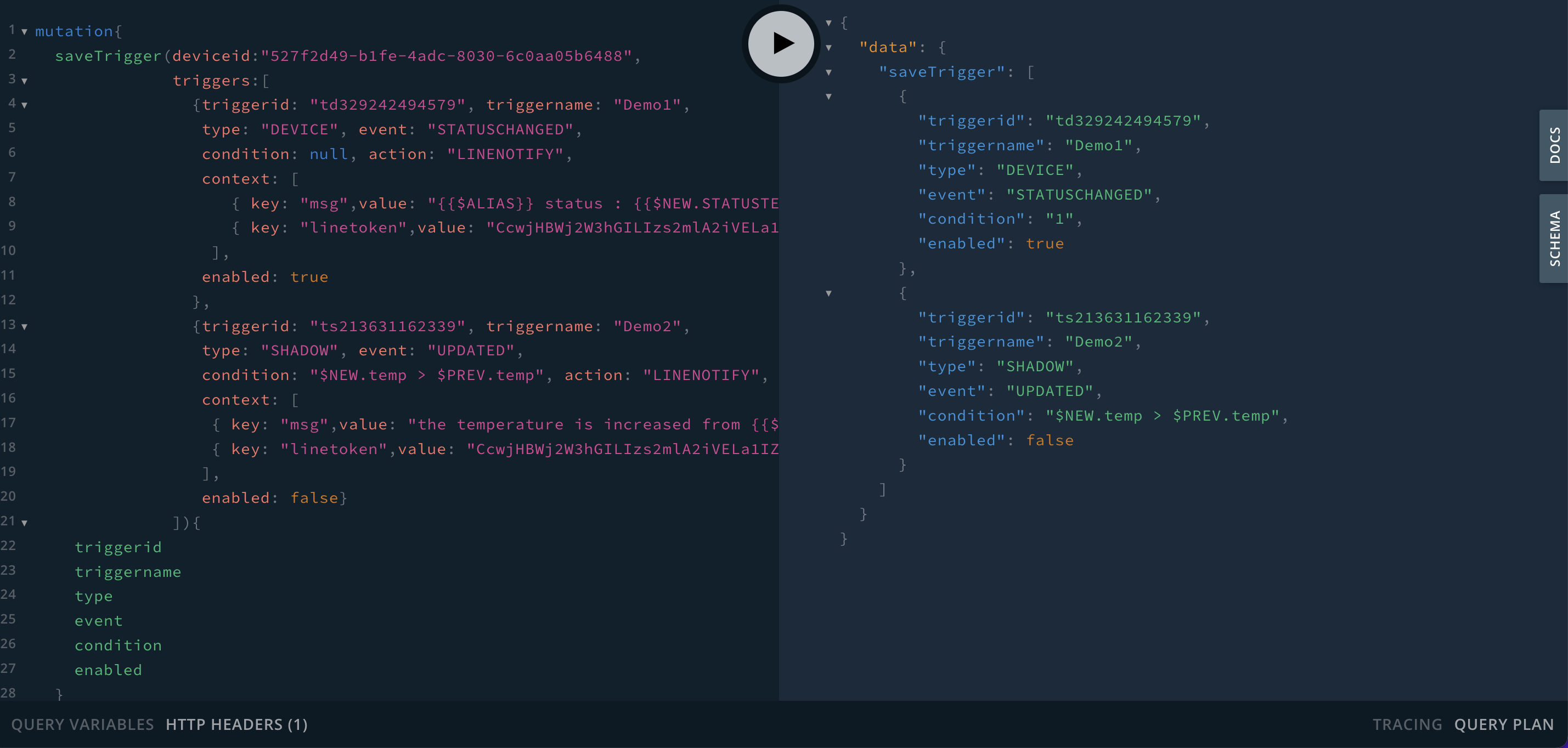
UnGroupDevice¶
To remove Devices from a Group, the details are as follows:ungroupDevice ( deviceid ):
Arguments
deviceid
: [String] is an Array of Device IDs to be removed from the group (required).
Query Variables
Authorization
: User Token
Response Type : [Device]
The response is an Array of Object Type Devices that were removed from the Group, consisting of:
alias
: String is the name of the Device.
createdtime
: Timestamp is the date and time the Device was created.
credential
: Credential is data for verifying identity. Object Type credential consists of:
secret
: String is the Device's secret code for Authentication.
description
: String is a Device description.
deviceid
: String is the Device ID.
devicesecret
: String is the secret code of the Device.
groupid
: String is the ID of the Group that the Device is under.
groupname
: String is the name of the Group that the Device is under.
projectid
: String is the Project ID that the Device is under.
status
: Int is Device’s connection status to the platform (0
offline,1
online)
tag
: String is a single-valued Device tag.
hashtag
: [String] is a multi-valued Device tag.
tags
: Json is a Device tag in Key Value format.
enabled
: Boolean is the Device activation status (true
enabled [default],false
disabled).
banned
: Boolean is the status of being suspended from the system (true
suspended,false
not suspended)
An example is shown in the following figure:
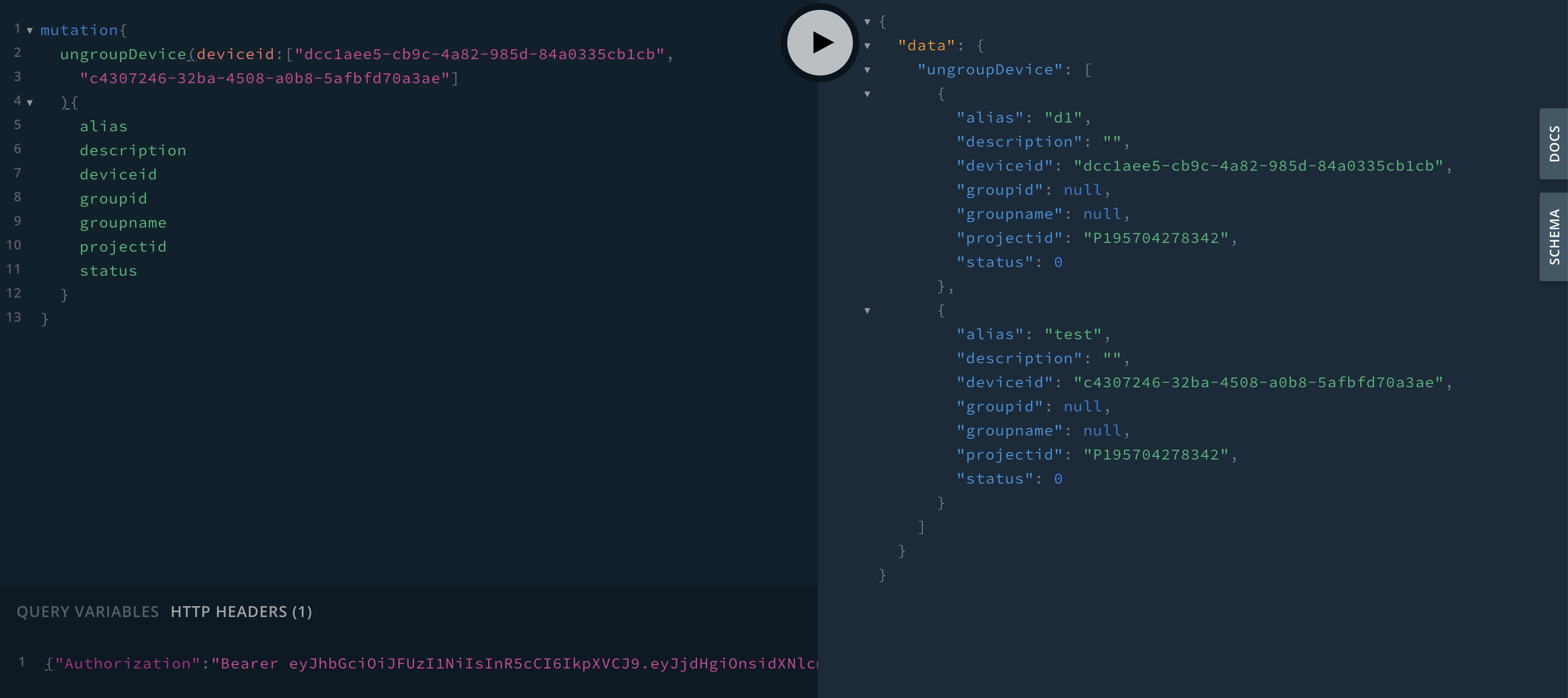
UpdateDevice¶
To edit general information of the Device, details are as follows:
updateDevice ( deviceid, projectid, groupid, tag, hashtag, tags ):
Arguments
deviceid
: String is the Device ID (required)
deviceinfo
: DeviiceInfo is an Object Type that contains details about the Device, including:
alias
: String is the name of the Device (if not specified it will be set to the same value as deviceid, used to refer to the Device).
description
: String is a Device description.
tag
: String is a single-valued Device tag.
hashtag
: [String] is a multi-valued Device tag.
tags
: JSON is a Device tag in Key Value format.
Query Variables
Authorization
: User Token
Response Type : Device
The response is Object Type Device consisting of
alias
: String is the name of the Device.
createdtime
: Timestamp is the date and time the Device was created.
credential
: Credential is data for verifying identity. Object Type credential consists of:
secret
: String is the Device's secret code for Authentication.
description
: String is a Device description.
deviceid
: String is the Device ID.
devicesecret
: String is the secret code of the Device.
groupid
: String is the ID of the Group that the Device is under.
groupname
: String is the name of the Group that the Device is under.
projectid
: String is the Project ID that the Device is under.
status
: Int is Device’s connection status to the platform (0
offline,1
online)
tag
: String is a single-valued Device tag.
hashtag
: [String] is a multi-valued Device tag.
tags
: JSON is a Device tag in Key Value format.
enabled
: Boolean is the Device activation status (true
enabled [default],false
disabled).
banned
: Boolean is the status of being suspended from the system (true
suspended,false
not suspended)
An example is shown in the following figure:
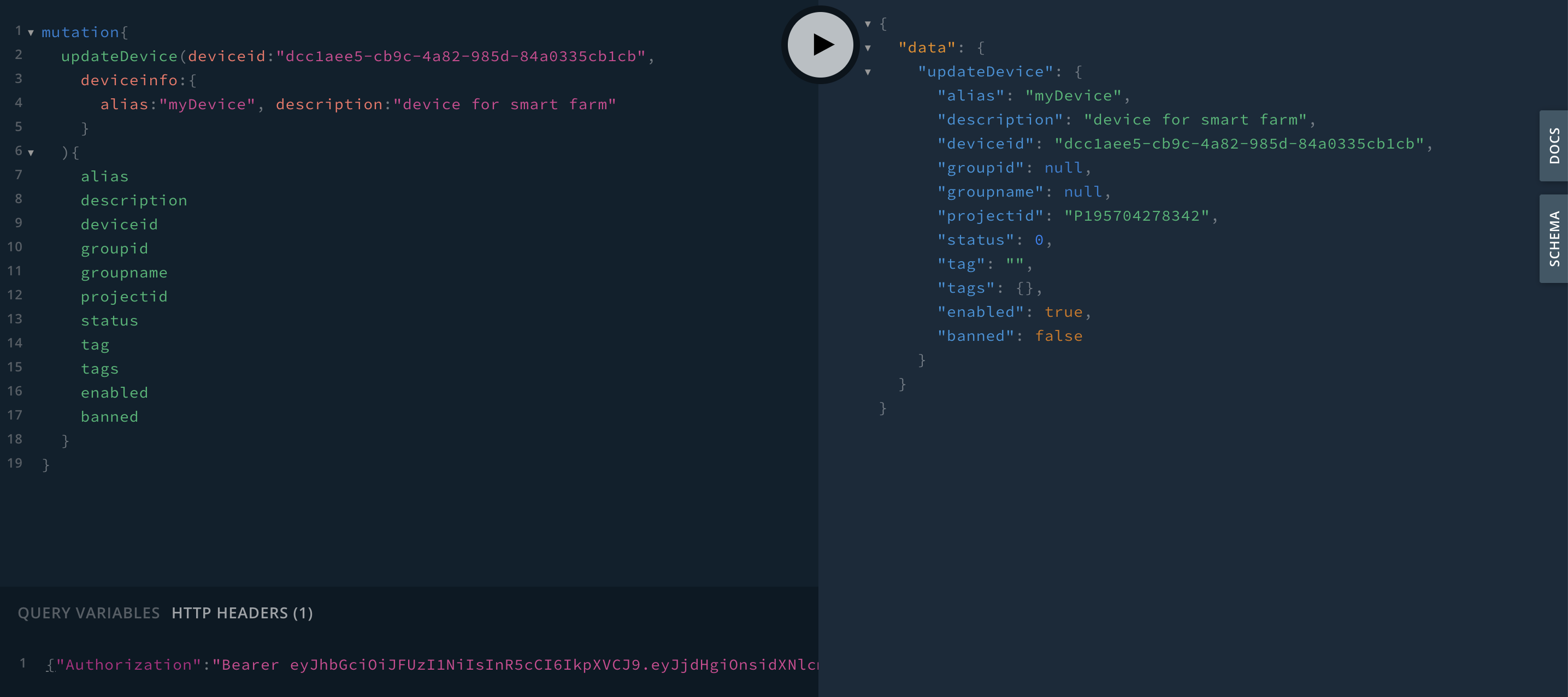
UpdateGroup¶
สTo edit general information of the Group, details are as follows:
updateGroup ( groupid, groupname, description, tag, hashtag, tags ):
Arguments
groupid
: String is the Group ID (required).
groupname
: String is the name of the Group to be edited.
description
: String is the description of the Group to be edited.
tag
: String is a single-valued Group tag.
hashtag
: [String] is a multi-valued Group tag.
tags
: JSON is a Group tag in Key Value format.
Query Variables
Authorization
: User Token
Response Type : Group
The response is an Object Type Group consisting of:
groupid
: String is the ID of the Group.
groupname
: String is the name of the Group.
projectid
: String is the Project ID.
createdtime
: Timestamp is the date and time the Group was created.
description
: String is a description of the Group.
tag
: String is a single-valued Group tag.
devicecount
: Int is the number of devices in the group.
hashtag
: [String] is a multi-valued Group tag.
tags
: Json is a Group tag in Key Value format.
An example is shown in the following figure:
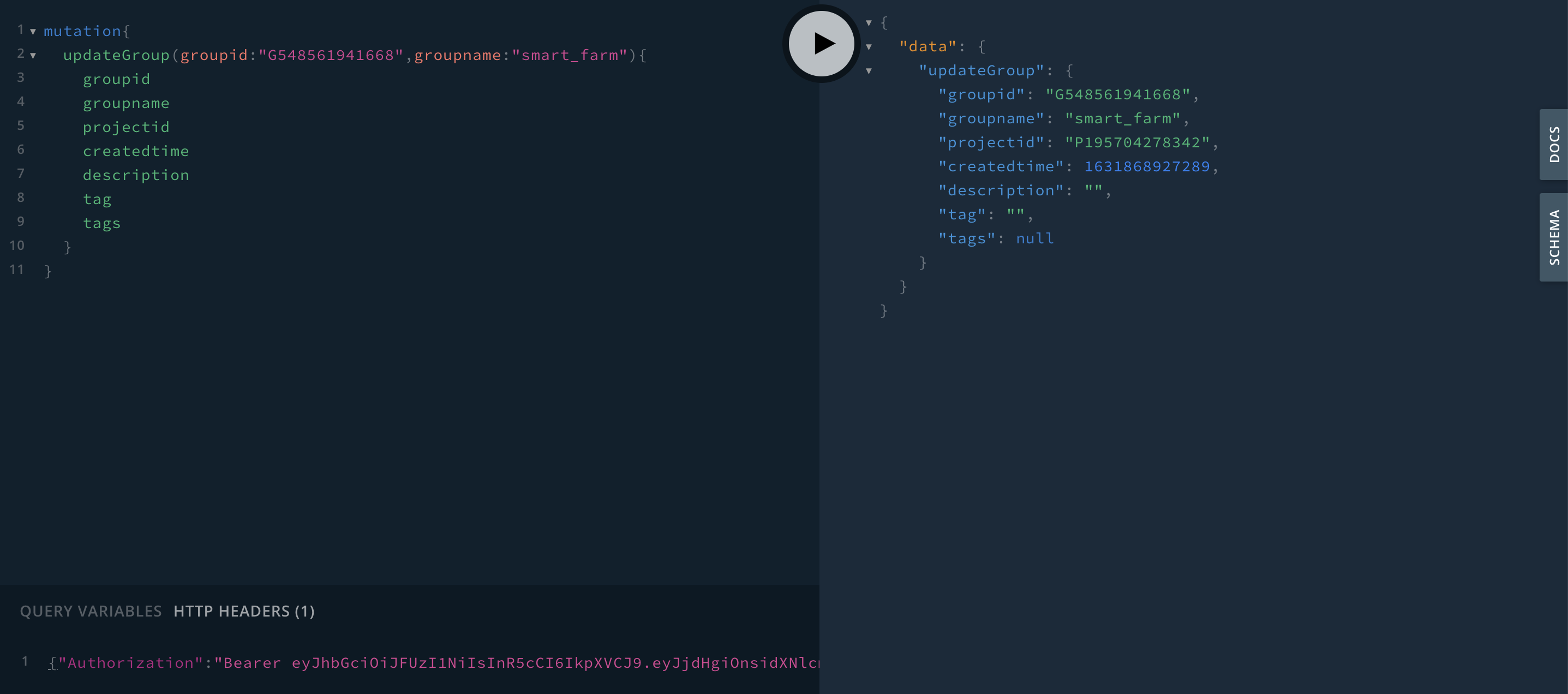
UpdateHook¶
To edit Event Hook information, details are as follows:
updateHook ( projectid, hookid, name, description, type, enabled, param ):
Arguments
projectid: String is the Project ID (required).
hookid
: String is the Event Hook ID (required).
name
: String is the name of the Event Hook (required).
description
: String is the Event Hook description.
type
: String is the state of the Event Hook (true
on,false
off).
enabled
: Boolean is the state of the Event Hook (true
enabled,false
disabled).
param
: JSON is the various parameters set in the Event Hook.
Query Variables
Authorization
: User Token
Response Type : Hook
The response is an Object Type Hook consisting of:
projectid
: String is the Project ID.
name
: String is the name of the Hook.
hookid
: String is the ID of Hook.
description
: String is a description of Hook.
type
: String is a type of Hook.
enabled
: Boolean is the state of the Event Hook (true
enabled,false
disabled).
param
: JSON is the various parameters set in the Hook.
An example is shown in the following figure:
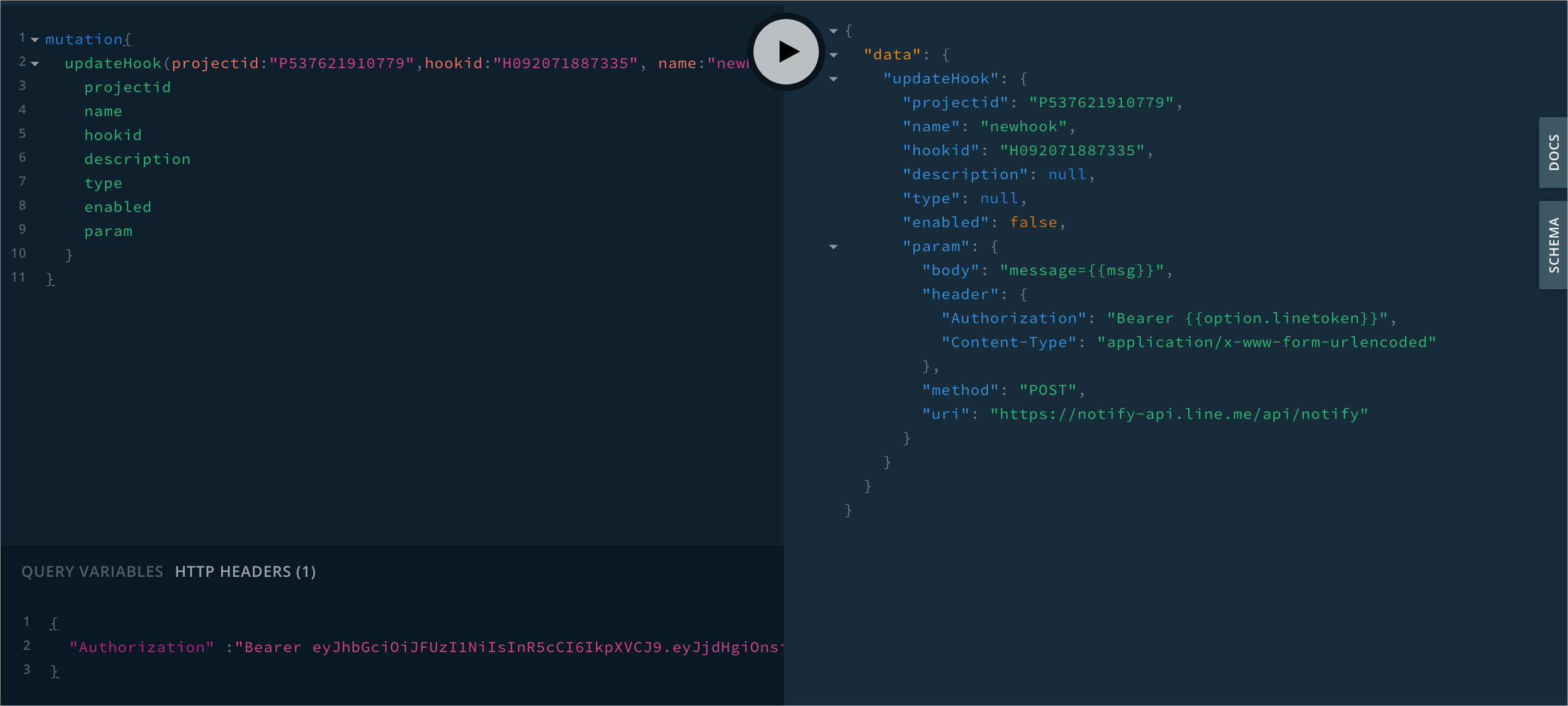
UpdateMembership¶
To edit Membership information, details are as follows:
updateMembership ( projectid, username, level ):
Arguments
projectid
: String is the Project ID (required)
username
: String is the username of the member user (required).
level
: UserLevel is the membership level, including (required) (See details Member)
Guest
Viewer
Editor
Master
Owner
Query Variables
Authorization
: User Token
Response Type : Membership
Response is Object Type Membership consists of
createdtime
: Timestamp is the date and time the Membership was created.
level
: UserLevel is the level of membership privileges. There are 5 levels in total (for details of privileges, see Member)
5
(Owner)
4
(Master)
3
(Editor)
2
(Viewer)
1
(Guest)
projectid
: String is the ID of the authorized project.
userid
: String is the user ID that is authorized.
username
: String is the username of the authorized user.
An example is shown in the following figure:
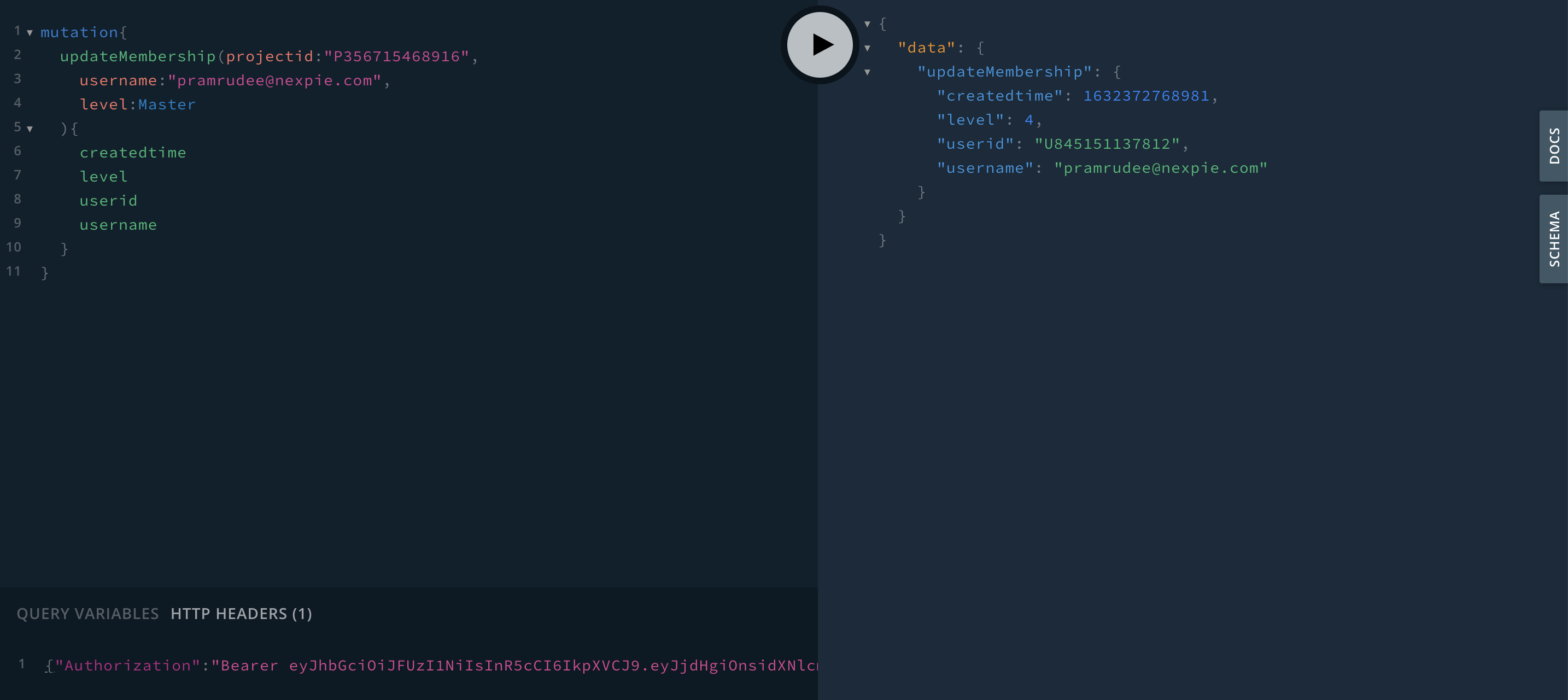
UpdateProject¶
To edit general information of the Project, details are as follows:
updateProject ( projectid, projectname, description, tag, hashtag, tags ):
Arguments
projectid
: String is the Project ID (required)
projectname
: String is the name of the Project.
description
: String is a project description.
tag
: String is a single-valued Project tag.
hashtag
: [String] is a multi-valued Project tag.
tags
: TagsInput is the project tags in Key Value format.
Query Variables
Authorization
: User Token
Response Type : Project
The response is Object Type Project consisting of:
projectname
: String is the name of the Project.
projectid
: String is the Project ID.
createdtime
: Timestamp is the date and time the project was created.
description
: String is a project description.
tags
: Json is a project tag in Key Value format.
tag
: String is a single-valued Project tag.
hashtag
: [String] is a multi-valued Project tag.
numberdevice
: Int is the number of devices under the project.
numbergroup
: Int is the number of Groups under the Project.
numberdeviceonline
: Int is the number of devices currently connected to the Platform under the Project.
numberdeviceoffline
: Int is number of Devices currently not connected to the Platform under the Project.
quota
: Quota is the status of each service with available quota, which includes:
apicall
: Boolean is a REST API service (true
available quota,false
unavailable or no quota)
connection
: Boolean คือ บริการเชื่อมต่อ Platform ของ Device (true
available quota,false
unavailable or no quota).
message
: Boolean is a Real Time Message service via MQTT Protocol (true
available quota,false
unavailable or no quota).
shadowops
: Boolean is a Shadow read/write service (true
available quota,false
unavailable or no quota)
store
: Boolean is a service that stores data in Time Series Data (true
available quota,false
unavailable or no quota)
trigger
: Boolean is Trigger & Action (Notification) service (true
available quota,false
unavailable or no quota)
datasource
: Boolean is the sum of the Byte size of the data that has been used by theapicall
service (true
available quota,false
unavailable or no quota)
An example is shown in the following figure:
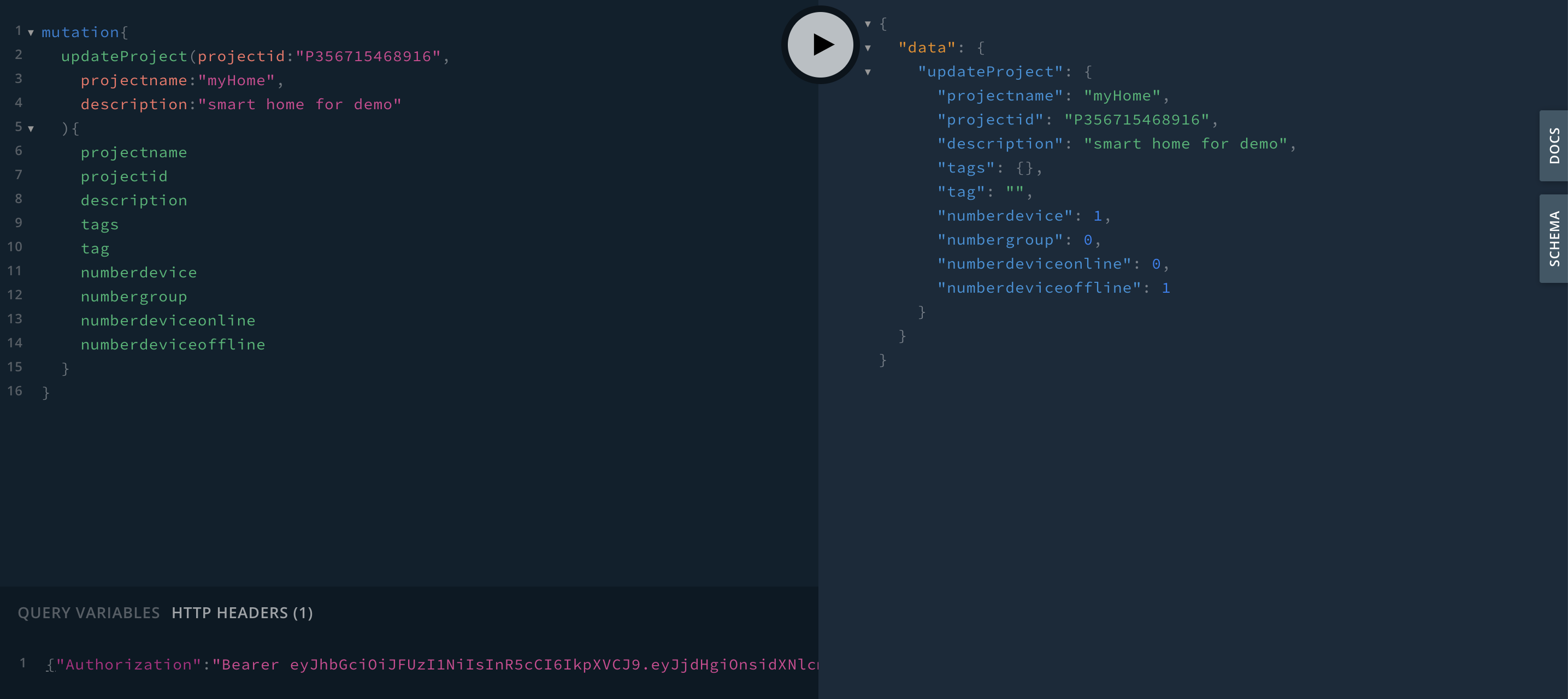
UpdateSchema¶
To edit Device Shadow Schema information, details are as follows:
updateSchema ( deviceid, value ):
Arguments
deviceid
: String is the Device ID (required)
value
: JSON is a defined Shadow Schema, which is in JSON format.
Query Variables
Authorization
: User Token
Response Type : Schema
The response is an Object Type Schema consisting of:
deviceid
: String is the Device ID.
value
: JSON is a defined Shadow Schema, which is in JSON format.
An example is shown in the following figure:
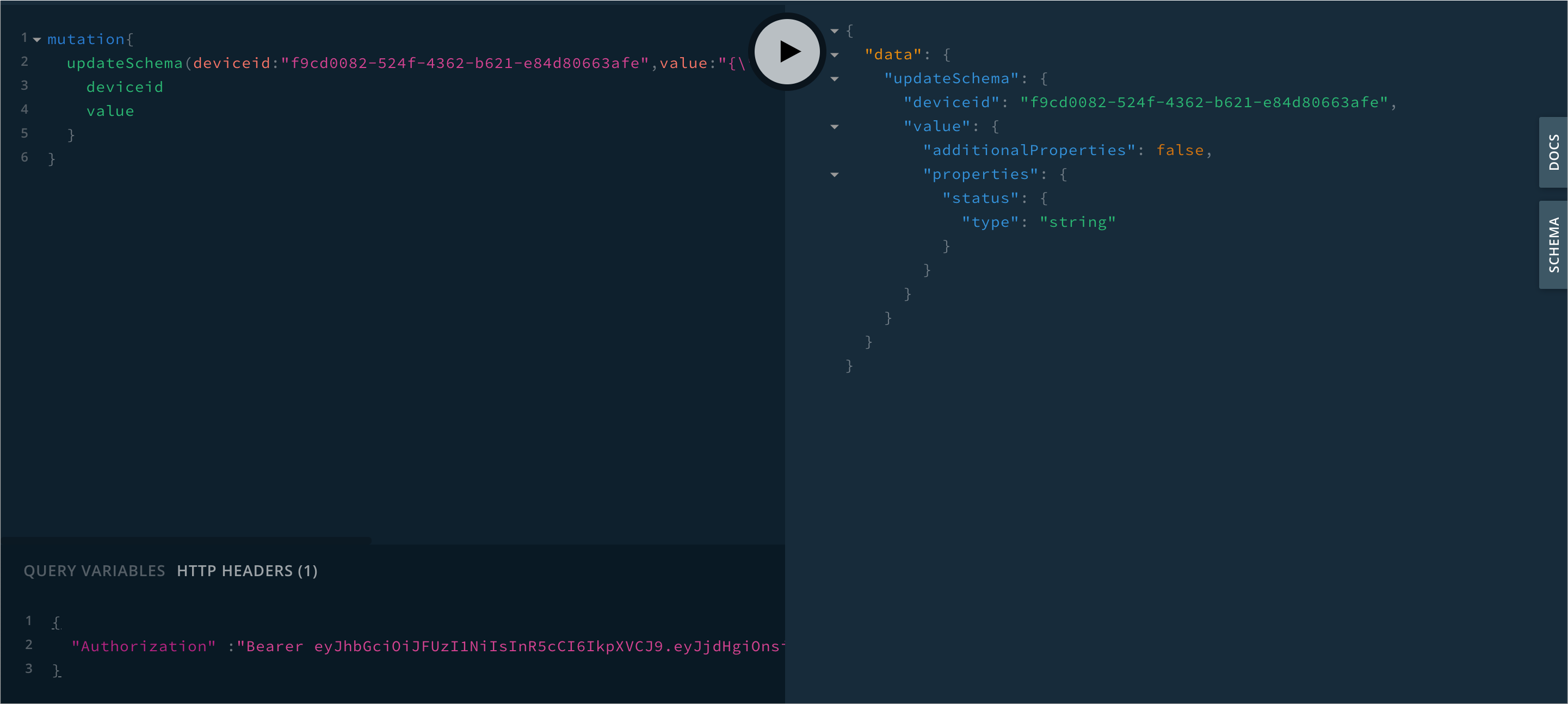
UpdateTrigger¶
To edit individual Trigger data, the details are as follows:
updateTrigger ( deviceid, triggerid, triggername, type, event, condition, action, context, enabled ):
Arguments
deviceid
: String is the Devcie code (required).
triggerid
: String is the Trigger code (required).
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String is the event that triggers (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
).
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state ( true`` enabled,false
disabled).
Query Variables
Authorization
: User Token
Response Type : Trigger
The response is an Object Type Trigger consisting of:
triggerid
: String is the Trigger code (required).
triggername
: String is the name of the Trigger.
type
: String is of Trigger type (DEVICE
,SHADOW
)
event
: String is the event that triggers (STATUSCHANGED
paired with Trigger typeDEVICE
,UPDATED
paired with Trigger typeSHADOW
).
condition
: String is the trigger condition.
action
: String is an Event Hook that will be processed when the Trigger occurs.
context
: [ActionVarType] is a Key-Value variable that will send values for use in the Event Hook.
enabled
: Boolean is the activation state (true
enabled,false
disabled).
An example is shown in the following figure:
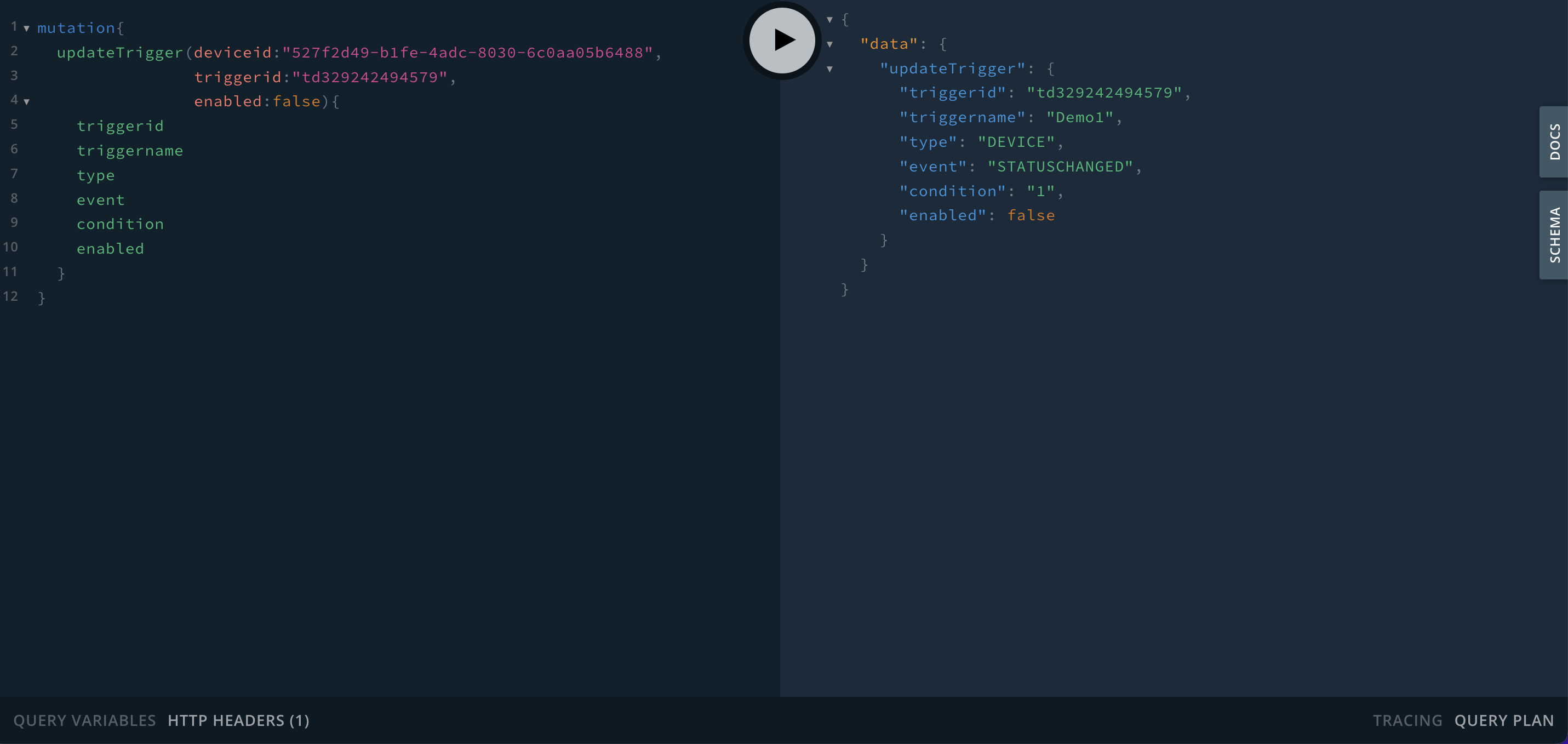
UpdateUserProfile¶
To edit general user information, details are as follows:
updateUserProfile ( fullname ):
Arguments
fullname
: String is the user's first name and last name.
Query Variables
Authorization
: User Token
Response Type : User
The response is Object Type User consisting of
username
: String is the username.
enail
: String is the user email.
profile
: Profile is an Object Type Profile consisting of:
fullname
: String is the first name and last name.
phonenumber
: String is a phone number.
organization
: String is the name of the department/organization/company that belongs to.
created
: String is the date and time of registration.
userid
: String is the User ID.
An example is shown in the following figure:
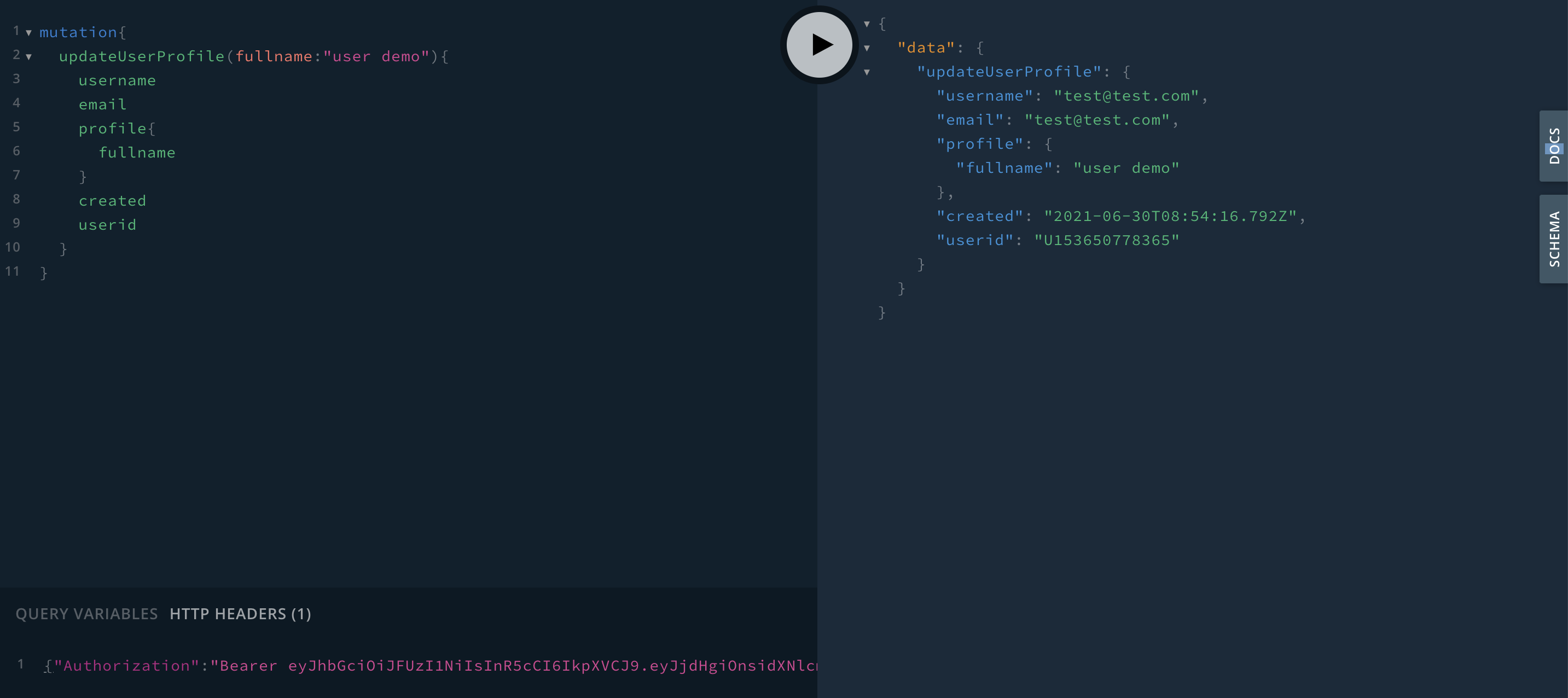
WriteShadow¶
To edit Device Shadow information, details are as follows:
writeShadow ( deviceid, data, timestamp, mode ):
Arguments
deviceid
: String is the Device ID (required)
data
: JSON is the Shadow you want to write, which will be in JSON format.
timestamp
: Timestamp is the date and time the data was changed (if not specified, the current time it was written).
mode
: WriteMode is the Shadow writing mode. There are 2 modes:
MERGE
is a merged shadow writing.
REPLACE
is an overwrite of shadow writing.
Query Variables
Authorization
: User Token
Response Type : Shadow
The response is Object Type Shadow consisting of
deviceid
: String is the Device ID.
data
: JSON is the defined Shadow, which will be in JSON format.
rev
: Int is the Revision number.
modified
: Timestamp is the date and time the data was last modified.
An example is shown in the following figure:
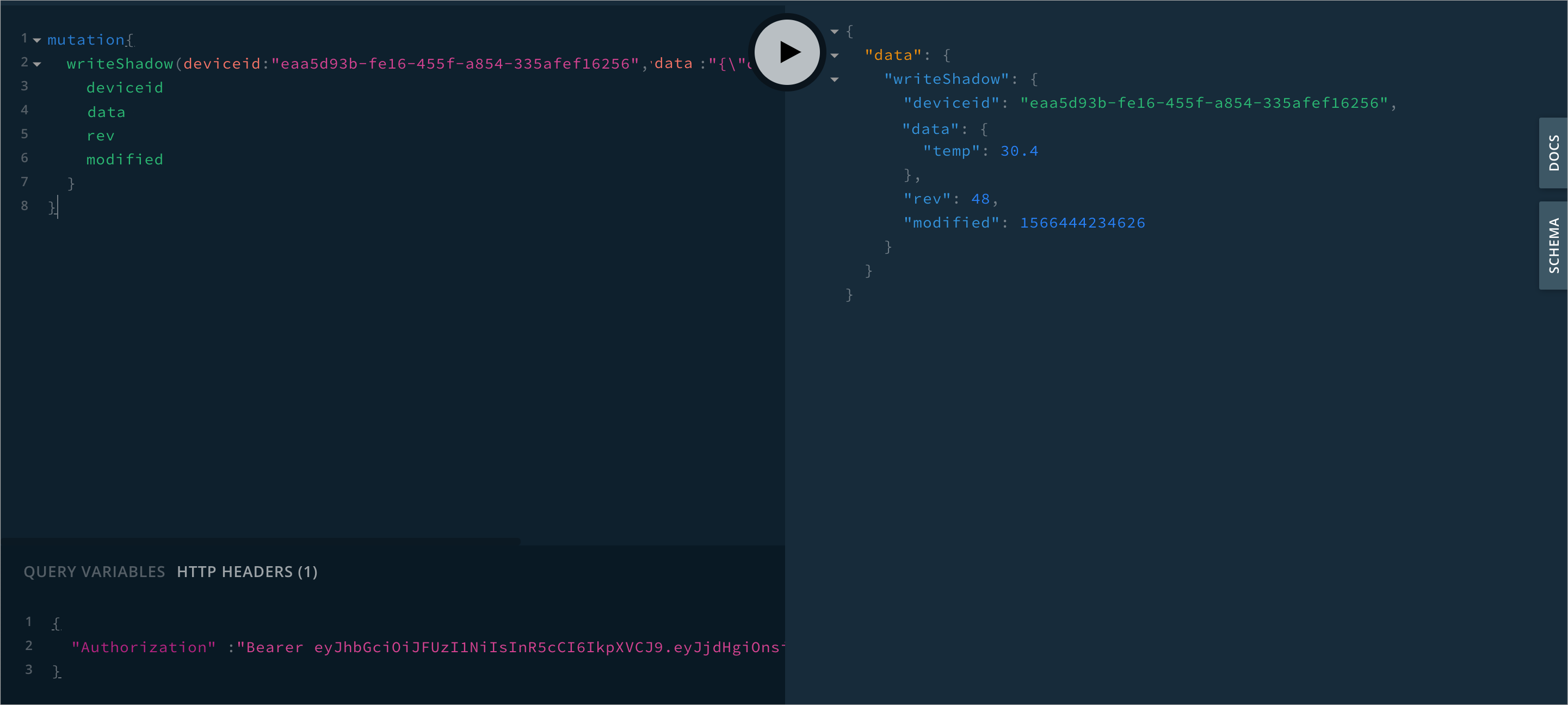
Note
Passing JSON Parameter Values
The values to be sent will be in JSON String format. For example, the values to be sent are:
json = { "body": "message={{msg}}", "method": "POST", "uri": "https://notify-api.line.me/api/notify" }
Convert to JSON String format to get:
string = "{ \"body\": \"message={{msg}}\", \"method\": \"POST\", \"uri\": \"https://notify-api.line.me/api/notify\" }"